How to create different type shape in android java
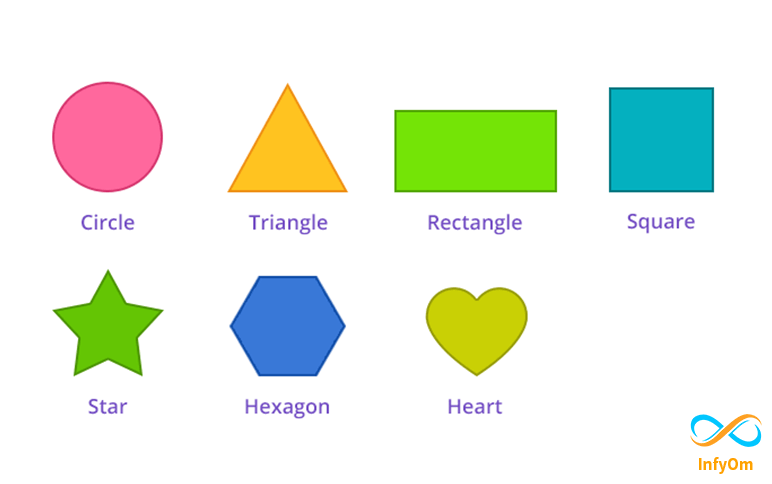
capsule shape,round shape,rectangle shape,square shape
How To Integrate onesignal push notification in Android
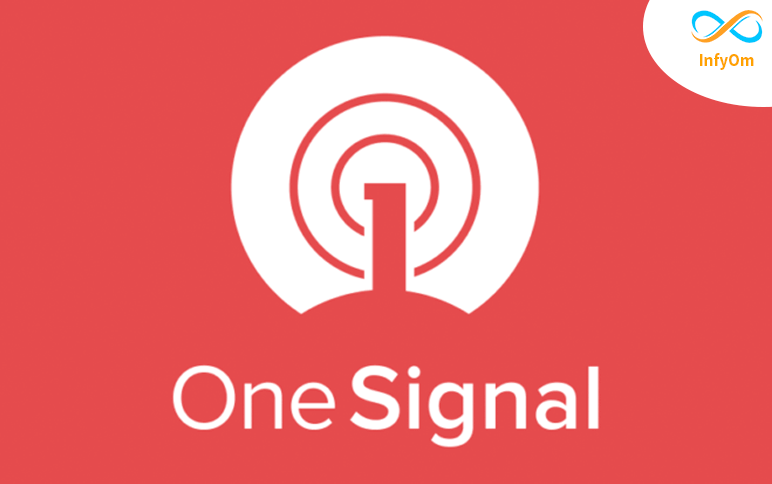
When you pick up your phone, the first messages you see push notifications maybe there’s a breaking news alert, game…
How to create any API response model class in android java
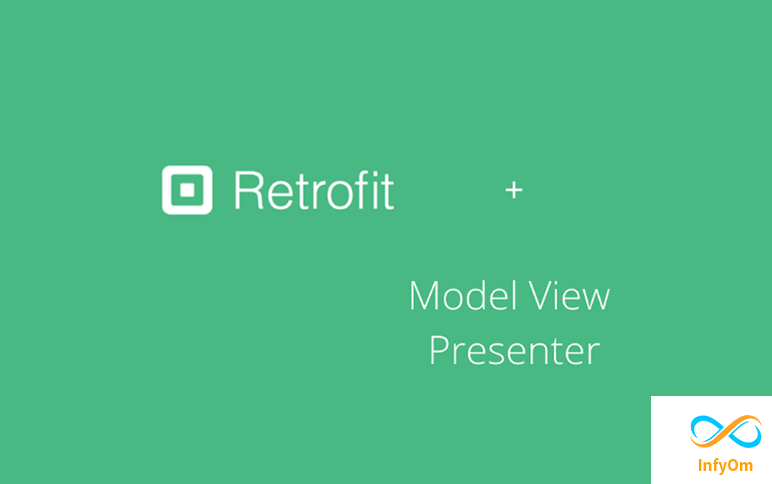
Any API data calling using a retrofit. Bulk data list gets and set over the android app.A very simple method explains…
Facebook Login With Firebase In Android Java
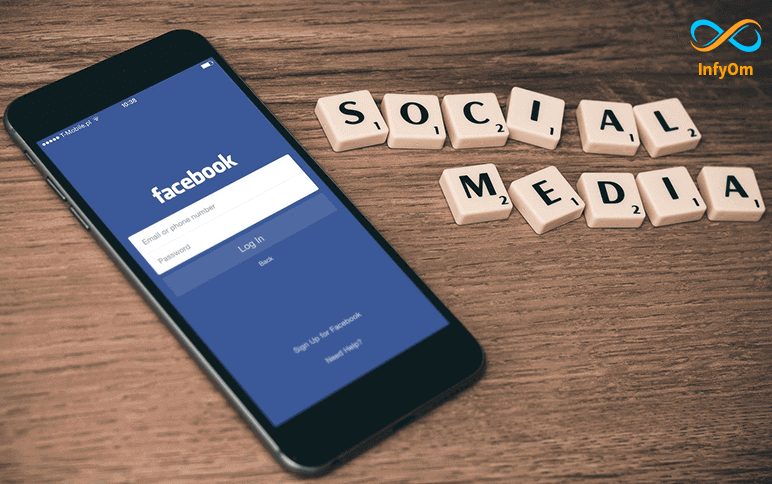
Facebook login with firebase app owner justify user login over app.some time user data upload over app in so necessary…
Send Device-to-Device Push Notification using Firebase Cloud Messaging
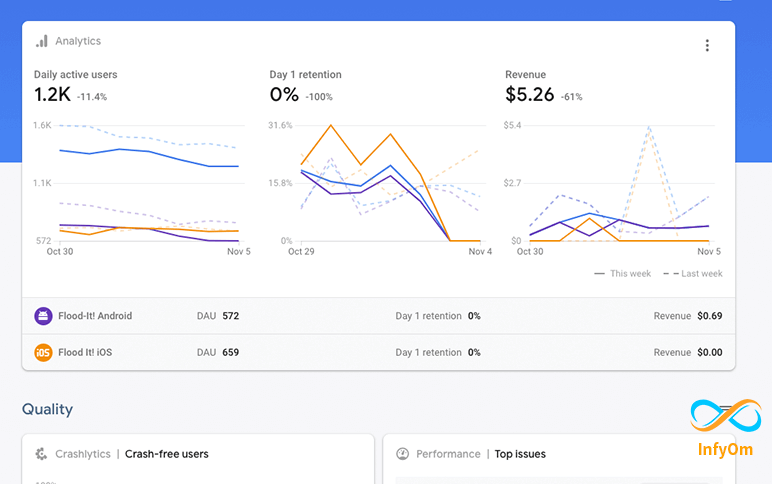
Firebase Cloud Messaging (FCM) is a cross-platform messaging solution that lets you deliver messages for…