How to Connect Gatsby to WordPress
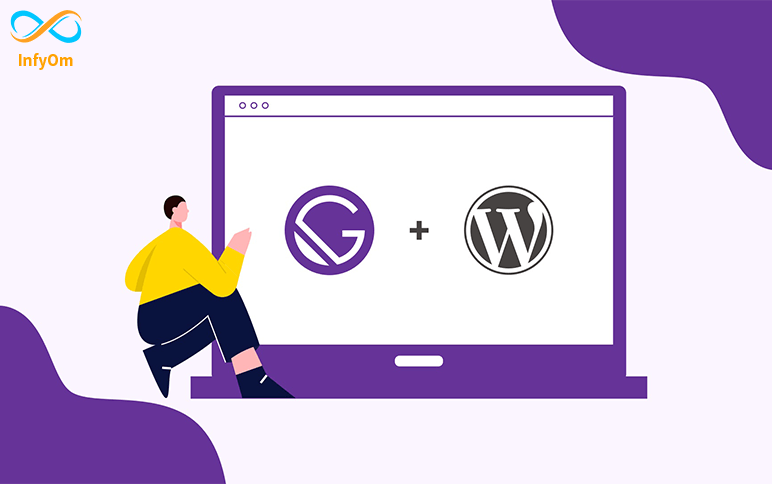
gatsby with wordpress
How to connect contentful CMS in the gatsby website
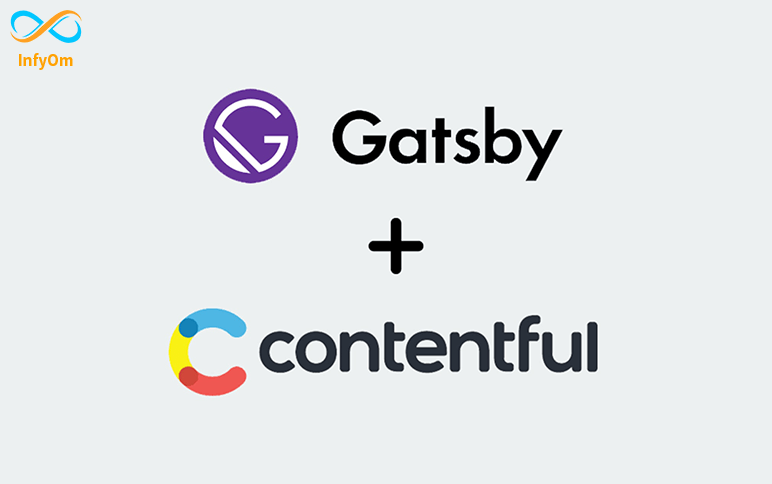
connect contentful CMS in the gatsby website
How to make a Progressive Web App
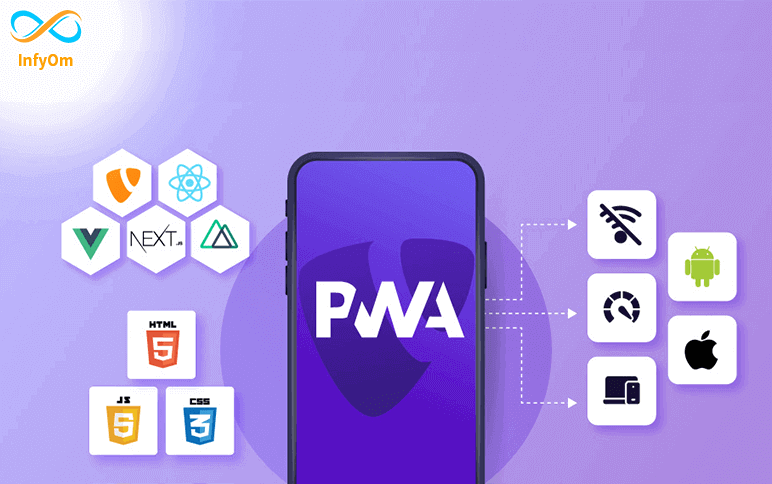
Create a PWA (Progressive Web App) website
Fix 404 while reloading Gatsby Website for dynamic client-only route
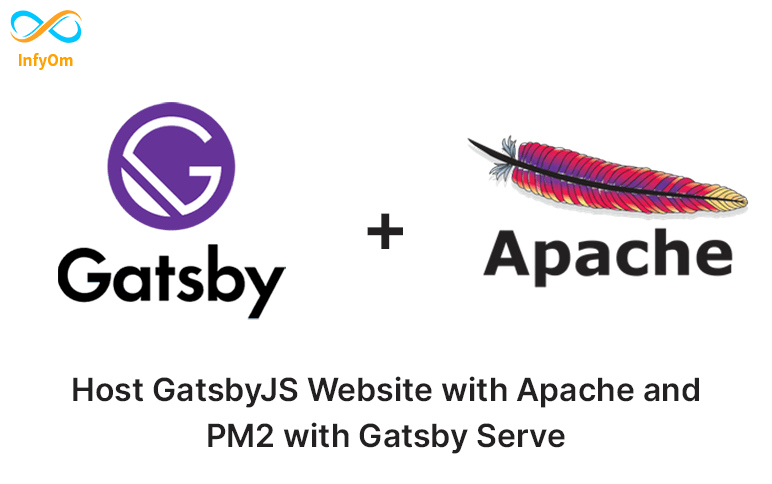
How to fix 404 page error while reloading a gatsby website which used dynamic cilent only routing hosted with Apache…
How to implement Google Analytics into Gatsby Site
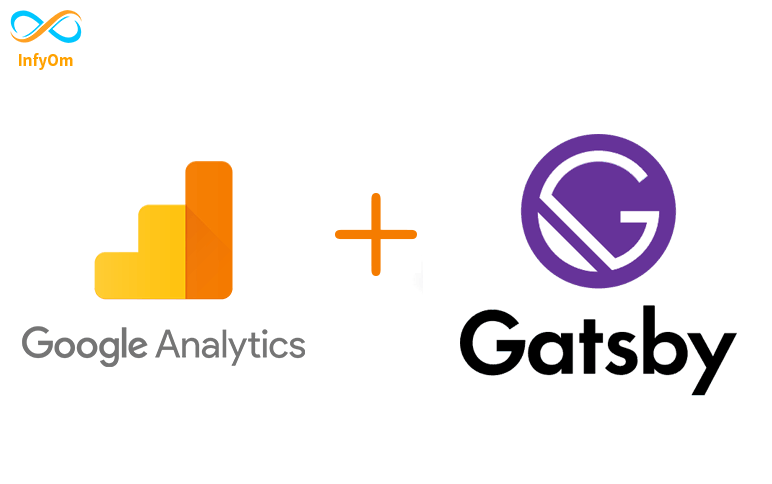
How to implement Google Analytics into Gatsby Site
How to load dynamic blog in Gatsby Site
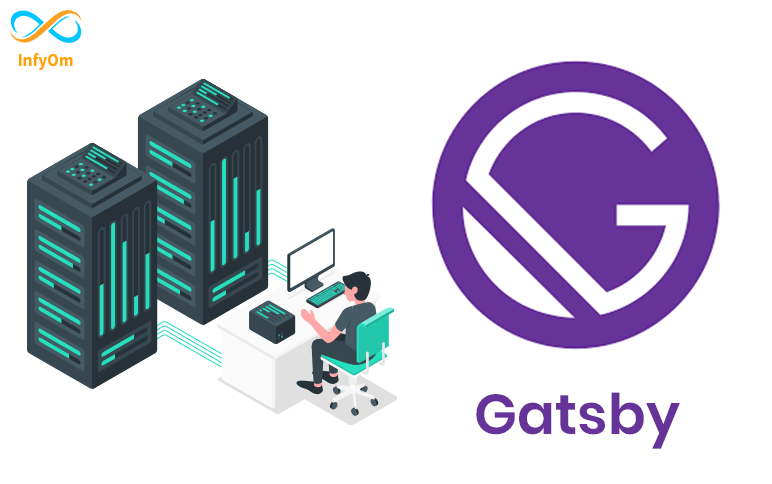
How to create a static blog page using API on Gastby
How to implement Mailchimp into Gatsby Site
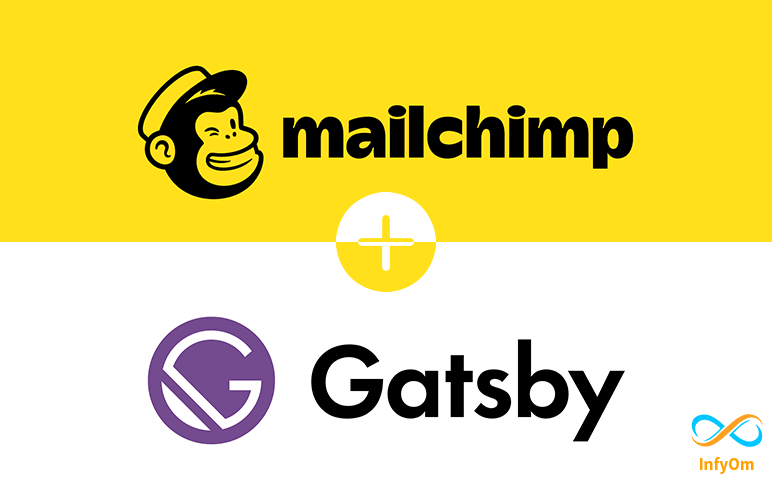
How to implement Mailchimp into Gatsby Site
How create a sitemap for your Gatsby site
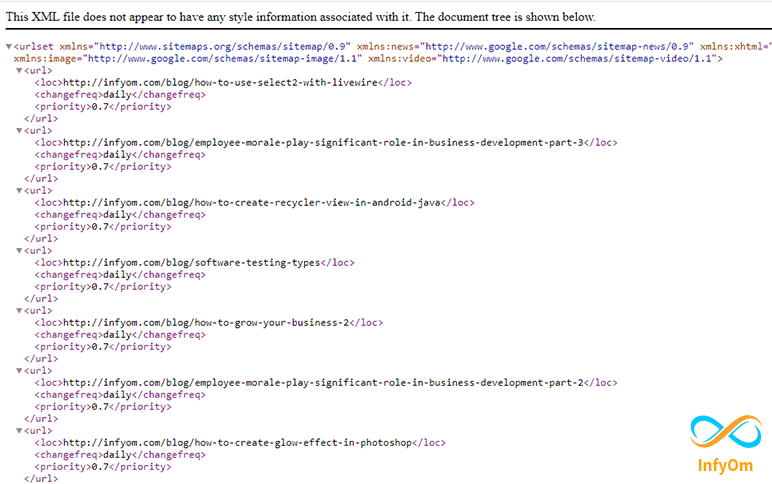
How create a sitemap for your Gatsby site in react