How to Setup Global Git Ignore in window
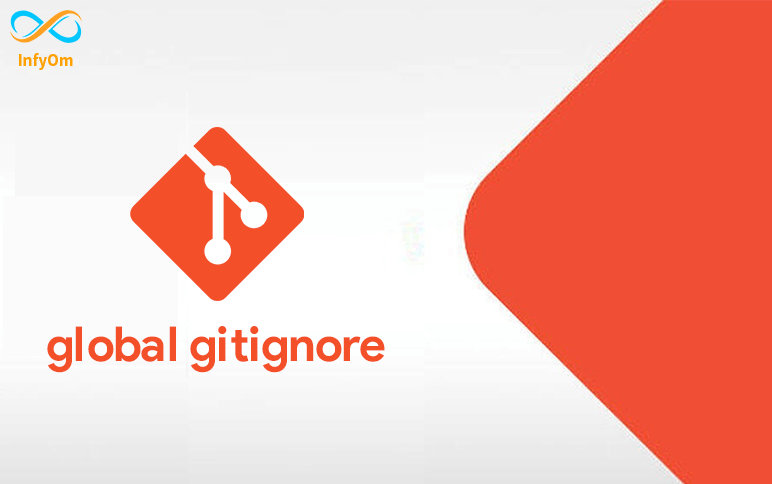
How to Setup Global Git Ignore
How to develop package into Laravel ?
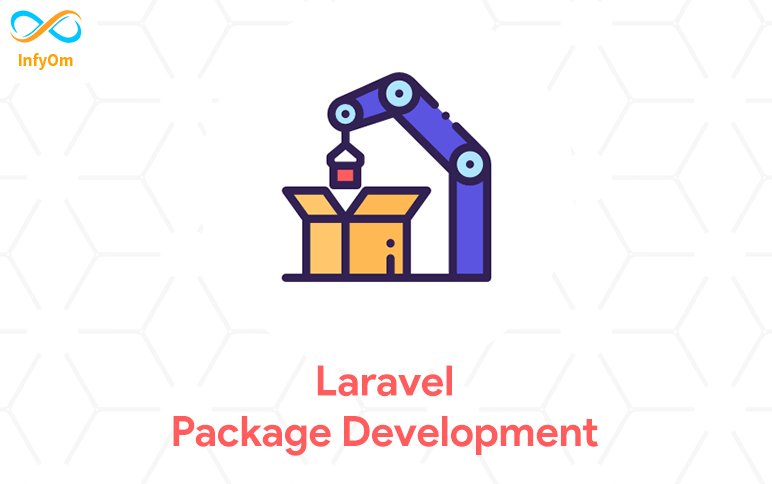
How to develop package by using laravel
Stisla Templates with JQuery Datatables
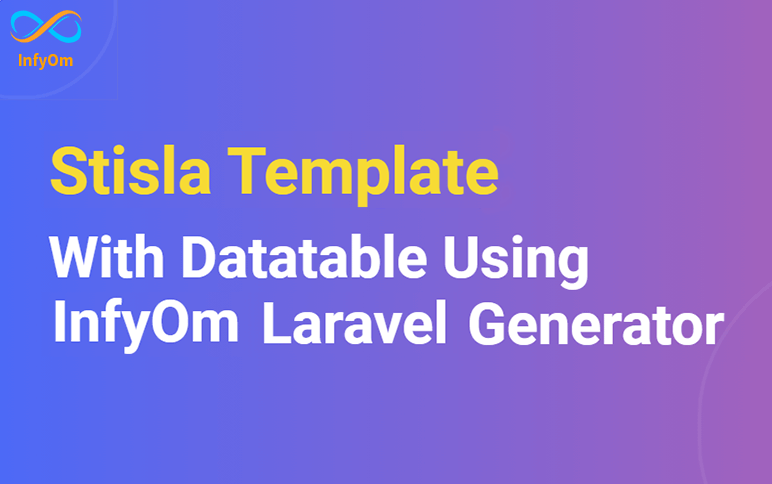
use stisla templates with JQuery datatables
How to create custom validation rules in Laravel ?
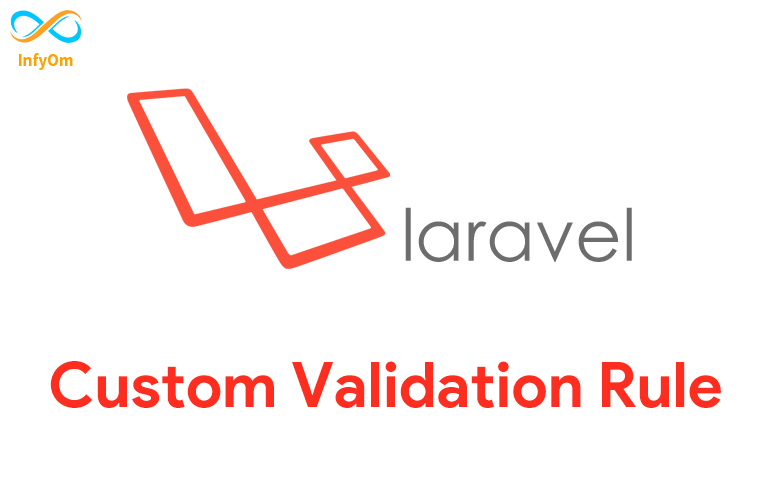
create custom validations rules in larave
Send real time notification with Pusher using Laravel and Javascript
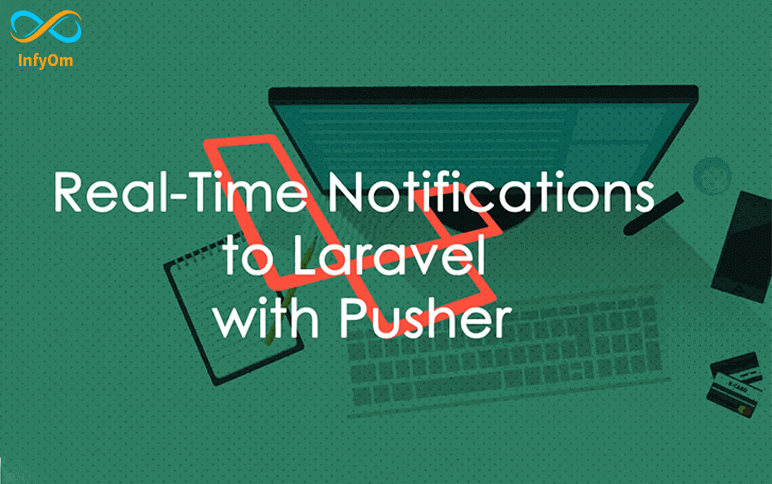
Send real time notification with Pusher using Laravel and Javascript
How to do payments with stripe checkout
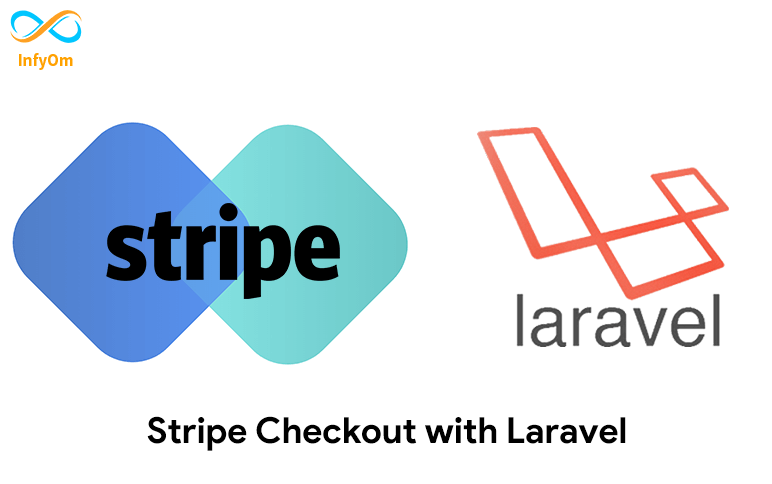
Do payments with stripe checkout method
Show saved annotations from database in document using PDFTron
Here we will learn how to import annotations saved in the database using PDFTron. In my last blog, we have learned how…
How to generate thumbnails by using Spatie Media Library
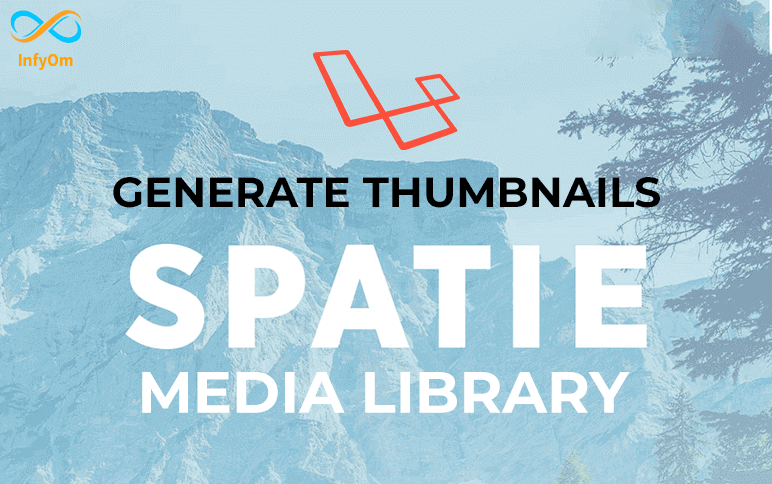
Generate thumbnails using laravel spatie media library
How to integrate Zoom Meeting APIs with Laravel
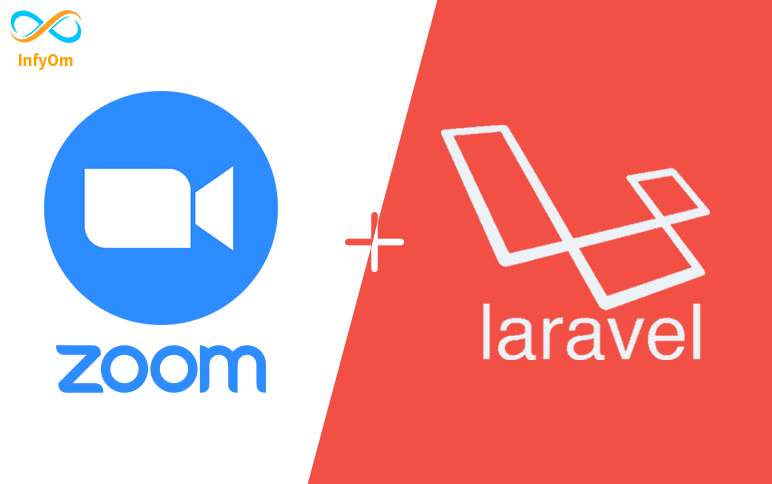
laravel, zoom meeting, zoom api, laravel zoom meeting
Make fully configurable livewire searching component
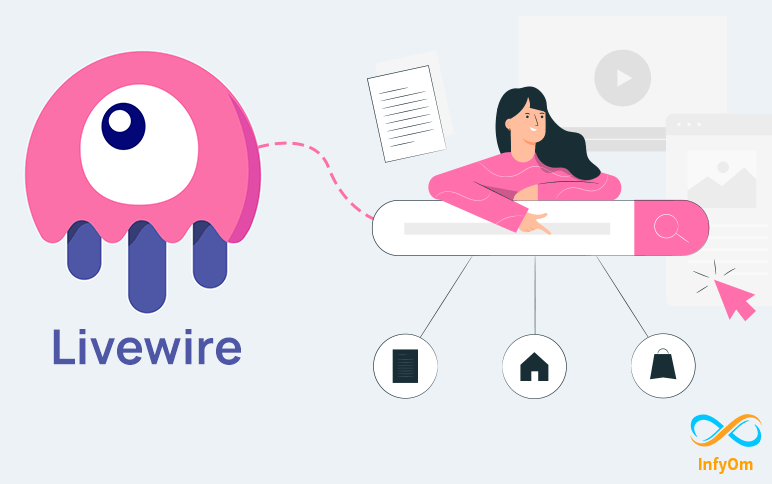
laravel. livewire, livewire searching, livewire component