[Best-Practices] Securing NodeJS Express APIs with JWT Authentication and custom Authorization
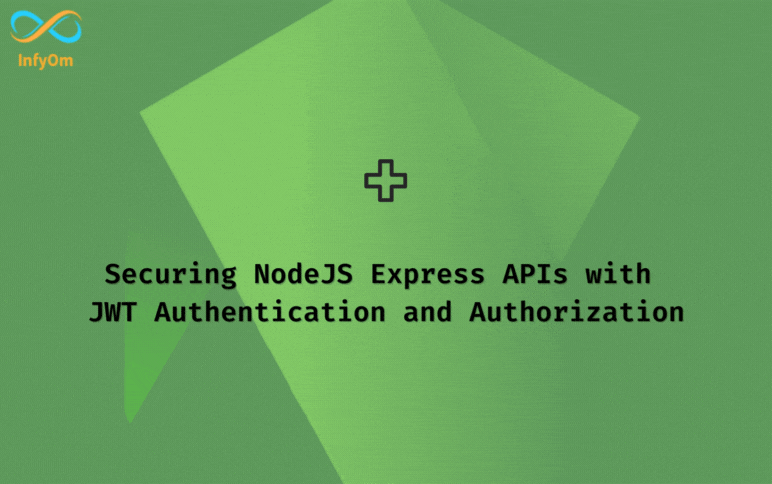
Securing the express API using JWT tokens and custom authorizations
Node.js vs. Deno: Which is better?
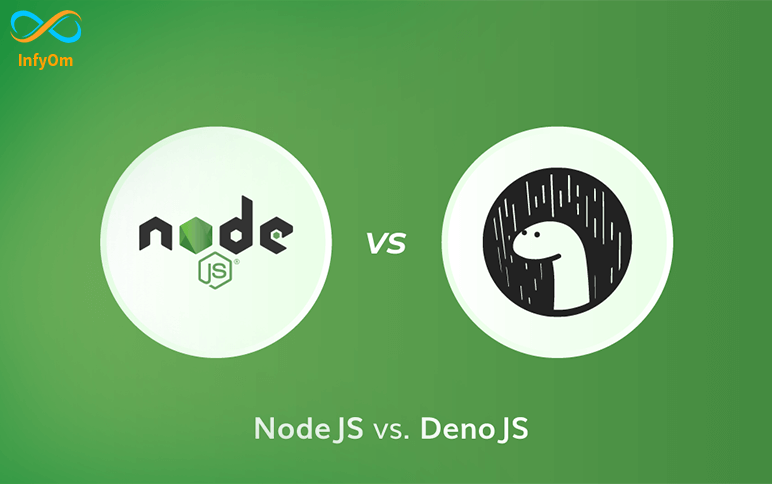
Which is better deno or nodejs? Will Deno replace node? Is Deno faster than node? Deno vs Node -…