Sometimes we need to load a large amount of data into memory. Like all the models we have in the database.
For e.g. PDF Printing, Perform some global updates, etc.
So the general practices we use in Laravel is to write the following code,
$users = User::all();
Just imagine I have 10,000 users in the database and when I load all the users in one shot.
But it takes a really high amount of memory to load all the records and prepare Laravel Model class objects. And sometimes we also load them in chunks to save the memory, but in some use cases, chunking can not be the option.
Here is the screenshot of mine when I load 10,000 users into memory with the above code.
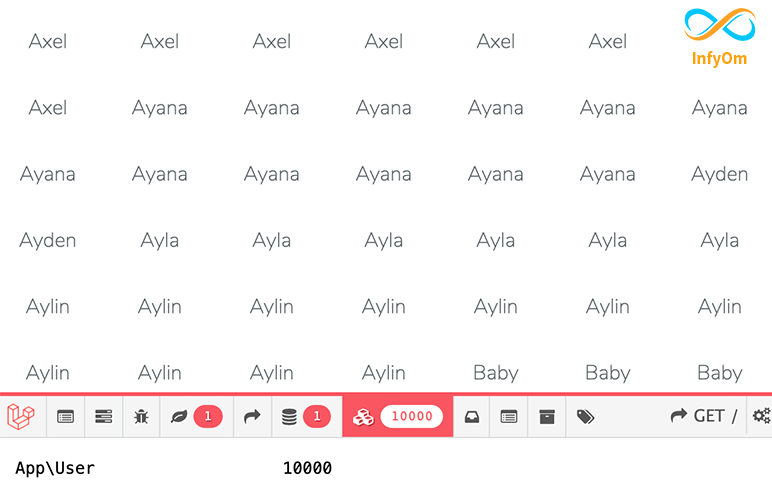
It’s using 37MB memory. Also, imagine the required memory if we are loading some relationships as well with these 10,000 records.
The Eloquent model is a great way to handle operations with lots of features like Mutators, Relationships, and much more.
But we really do not use these features all the time. We simply output or use the direct values which are stored in the table. So ideally, we do not need an eloquent model at all, if we are not going to use these features.
In those cases, Laravel also has a handy function toBase(). By calling this function it will retrieve the data from the database but it will not prepare the Eloquent models, but will just give us raw data and help us to save a ton of memory.
So my revised code will look something like this,
$users = User::toBase()->get();
Check the revised memory screenshot after adding the toBase function.
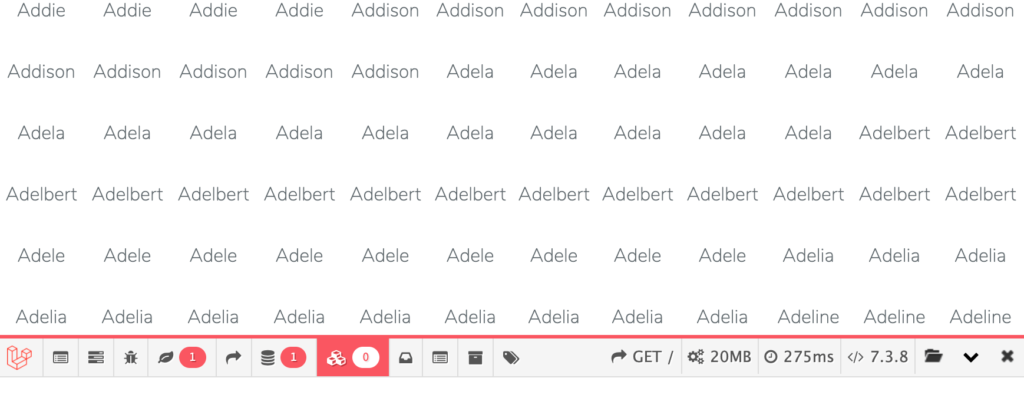
So it almost saves 50% of the memory. It’s reduced from 35MB to 20MB and the application also works much much faster, because it doesn’t need to spend time in preparing 10,000 Eloquent models.
So if you are not really going to use features of Eloquent and loading a large amount of data, then the toBase function can be really useful.
Here you can find a full video tutorial for the same.