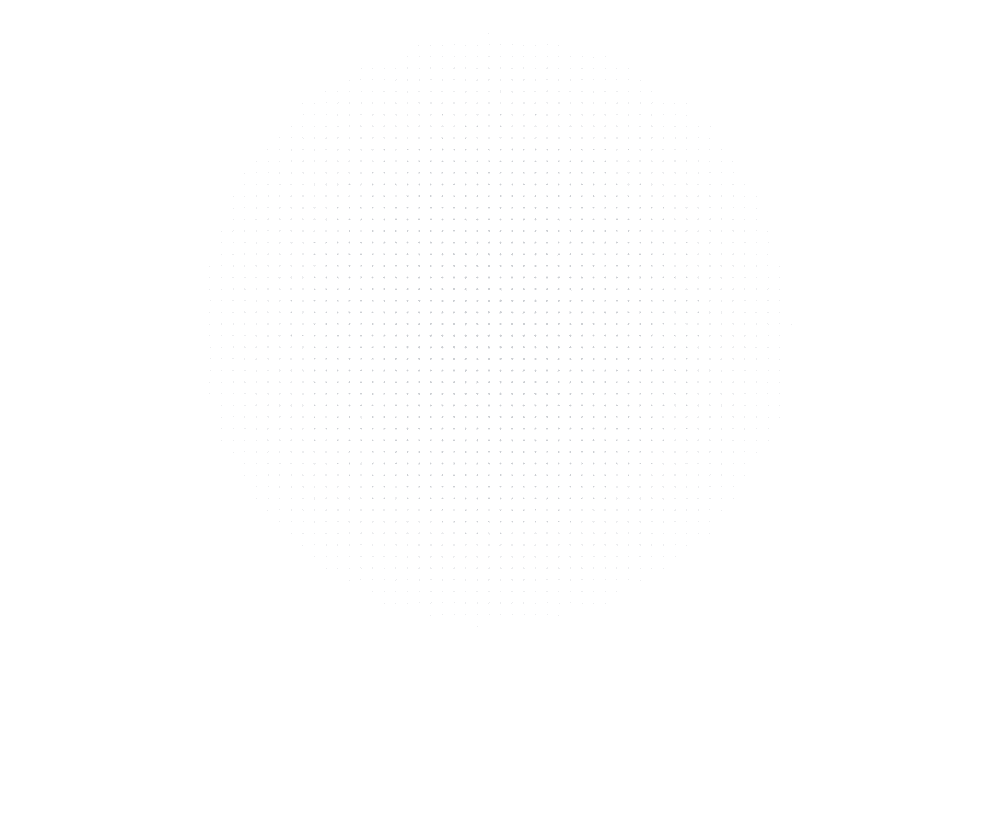
Latest Insights
Explore our blog for the latest trends, tips, tutorials and technologies that are shaping the future of software development.
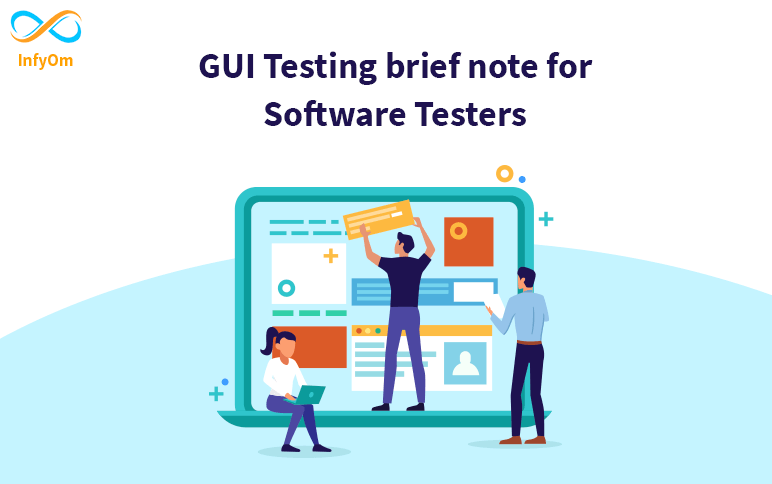
GUI Testing brief note for Software Testers
GUI testing brief note
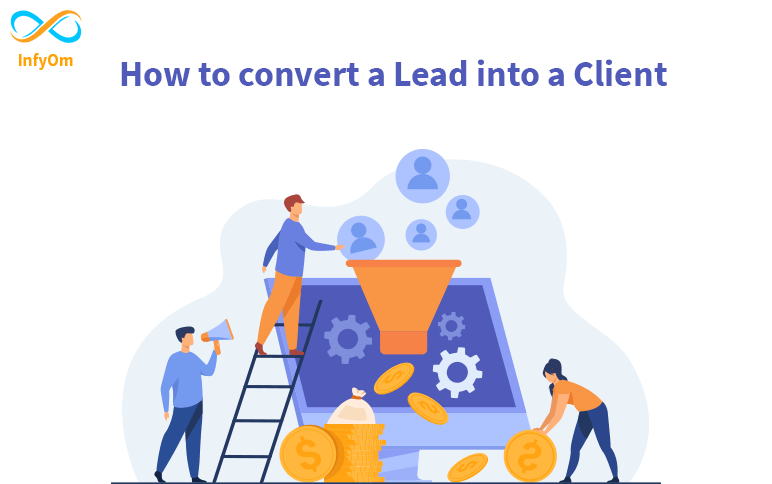
How to convert a Lead into a Client
Most of your leads through your conversion funnel will not automatically convert into…
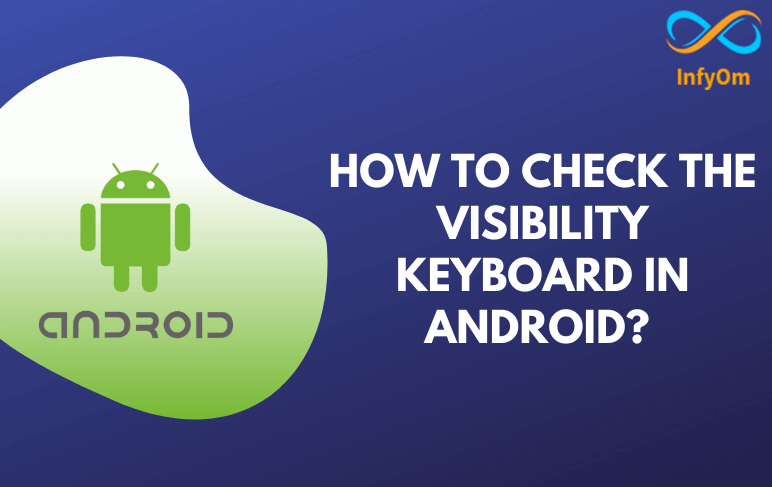
How to Check the Visibility Keyboard in Android?
The keyboard is one of the basic components of any Android application.
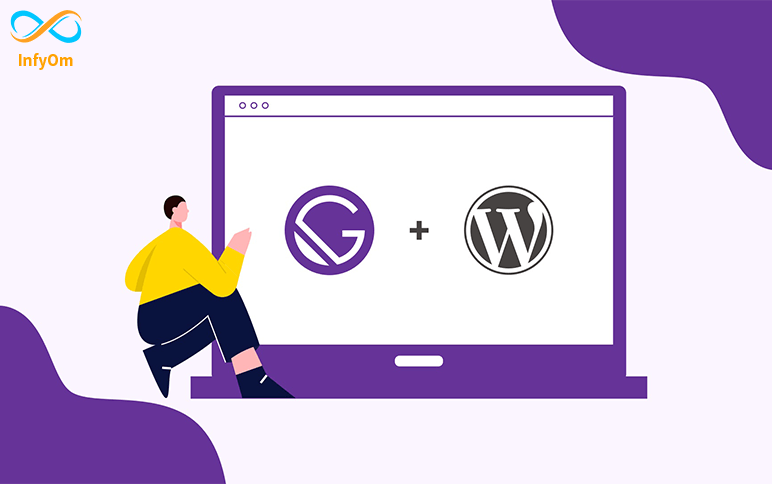
How to Connect Gatsby to WordPress
gatsby with wordpress
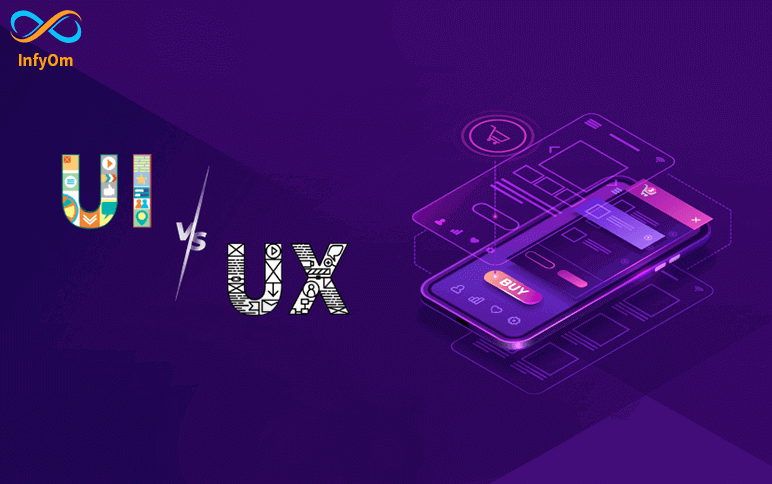
The Difference Between UX and UI Design
UX design is focused on anything that affects the user’s journey to solve that problem. UI design is focused on how the…
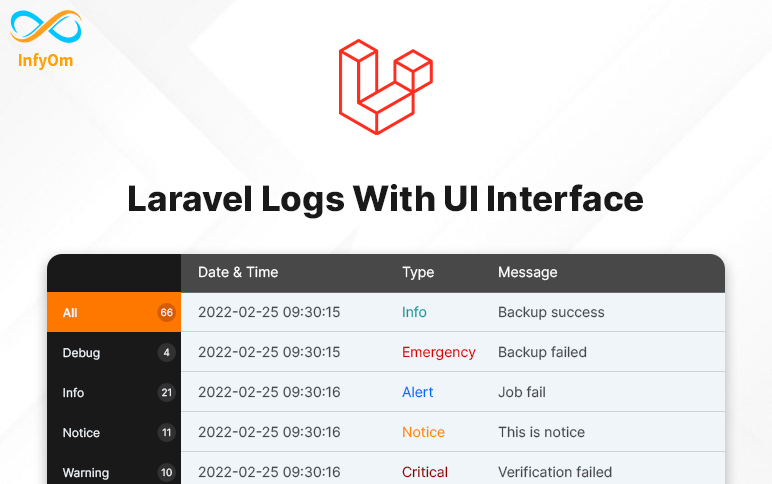
How to check Laravel logs with UI Interface?
Check laravel logs into UI Interface.
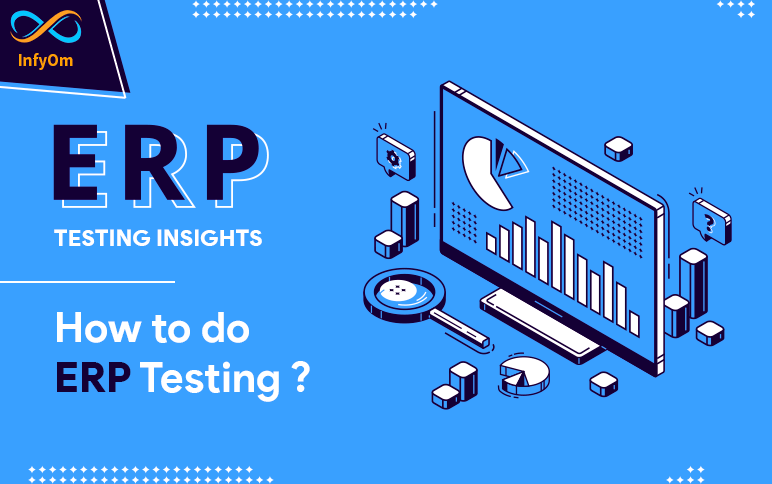
ERP Testing Insights: How to do ERP Testing ?
ERP Testing Insights
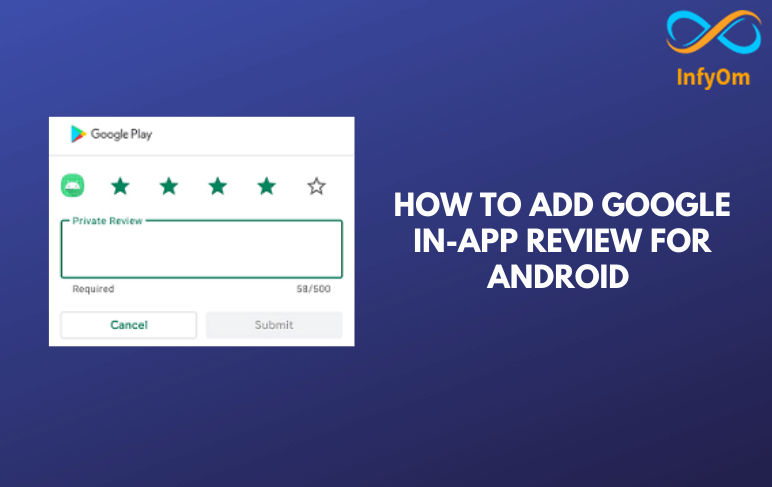
How To Add Google In-App Review for Android
The Google Play In-App Review API lets you prompt users to submit Play Store ratings and reviews without the…
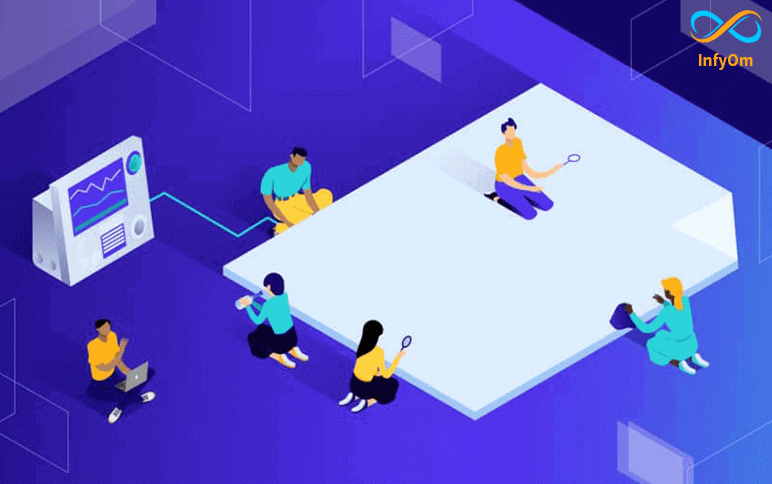
CSS NameSpacing ClassName
Namespacing can be used in any type of programming language, and the advantages are…