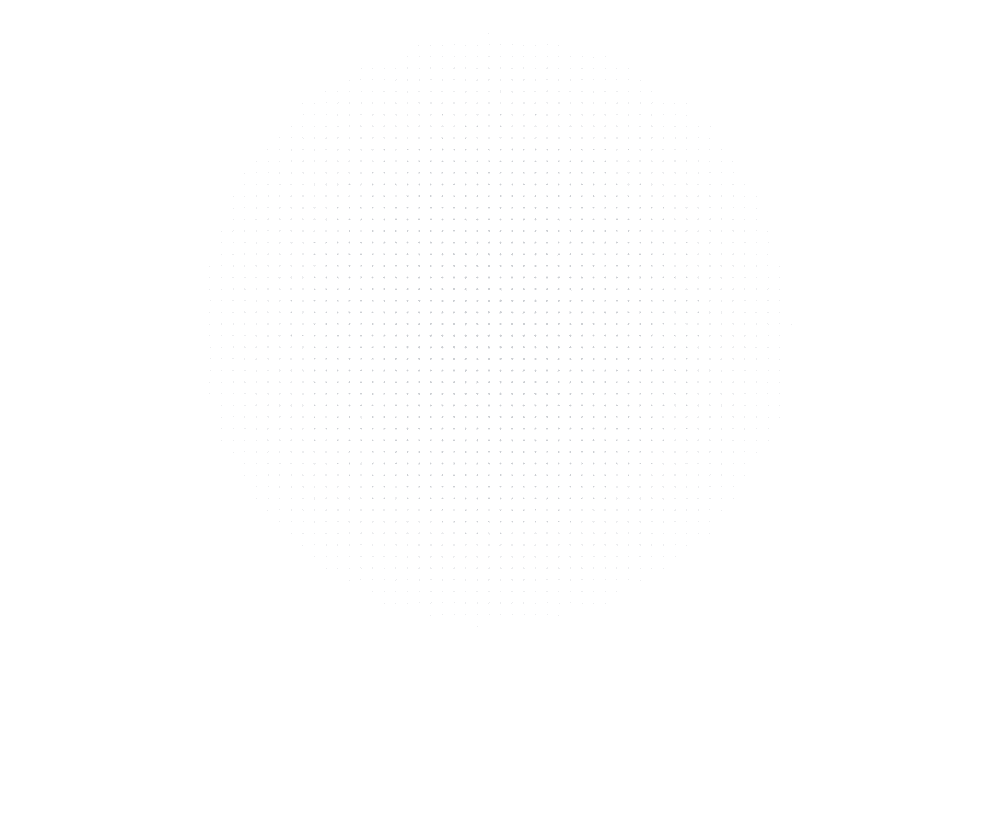
Latest Insights
Explore our blog for the latest trends, tips, tutorials and technologies that are shaping the future of software development.
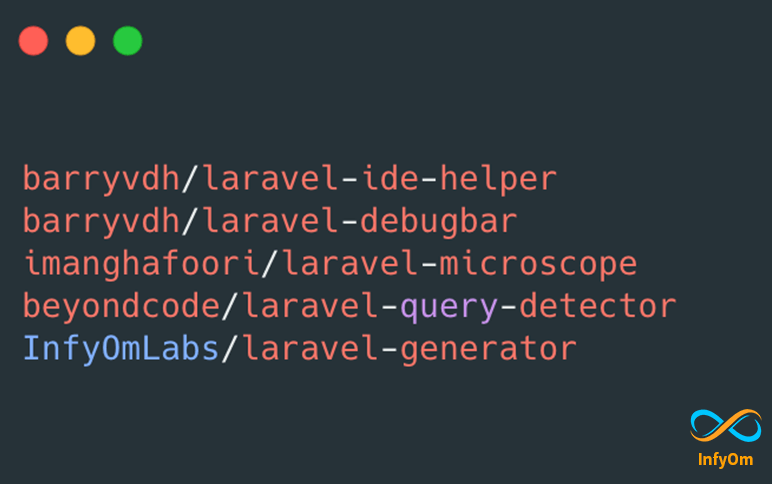
Laravel Packages we use everyday at InfyOm
List of laravel packages that we use every day in all of our laravel packages at…
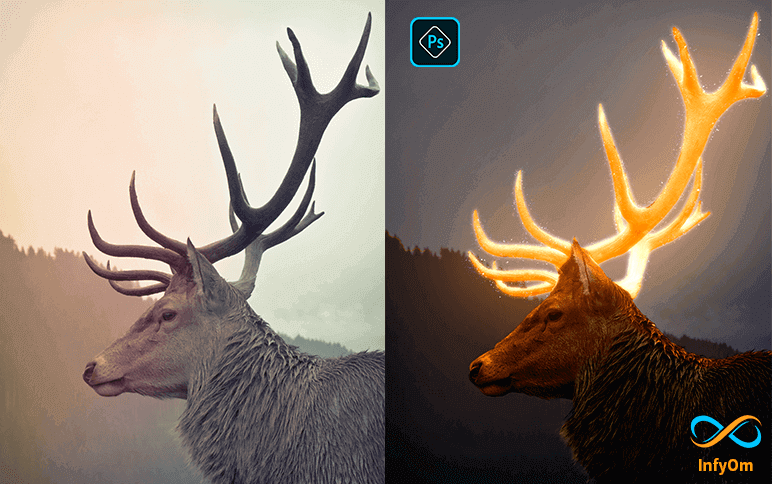
How to create glow effect in photoshop
In This Advanced Glow/Glowing Effect Photoshop Tutorial, learn How to make glowing effect in…
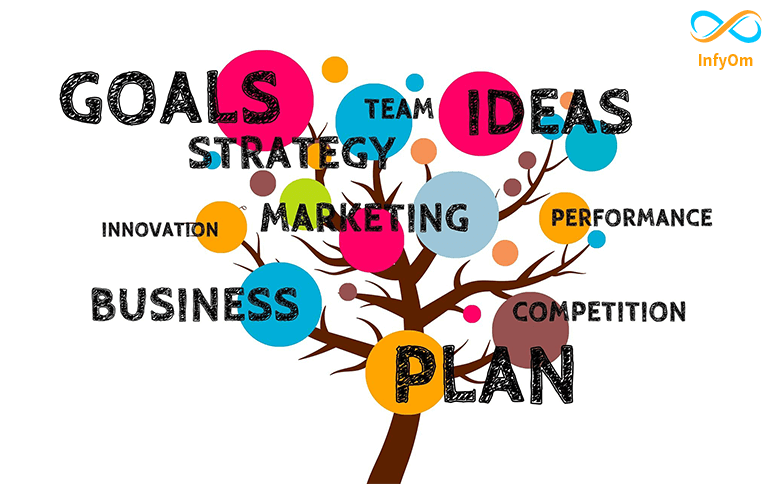
How To Grow Your Business – 1
One way to understand exactly what your customers want is through research and…
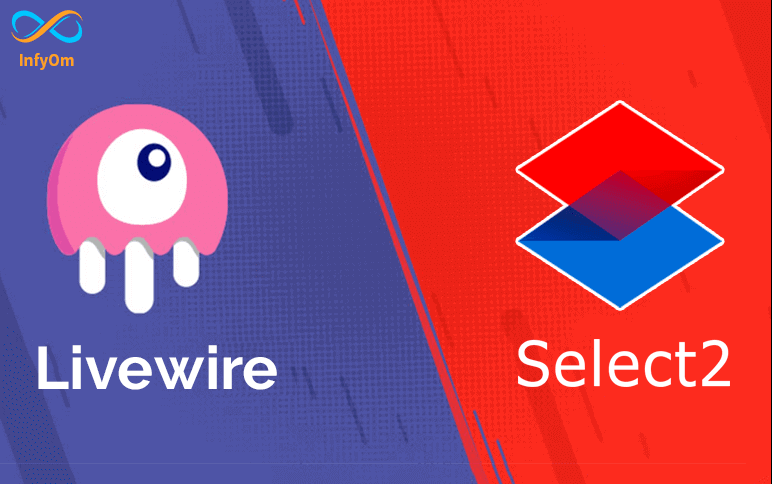
How to use select2 with livewire
livewire, select2, laravel
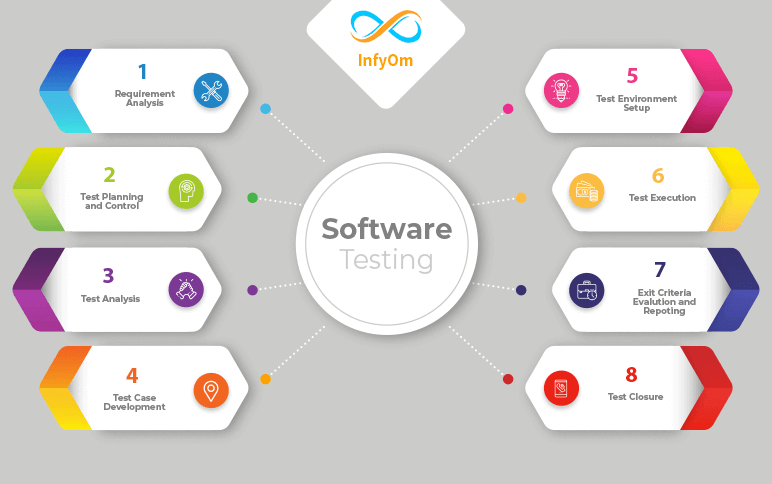
Software Testing Life Cycle
Software Testing Life Cycle. STLC is a sequence of different activities performed by the testing team to ensure the…
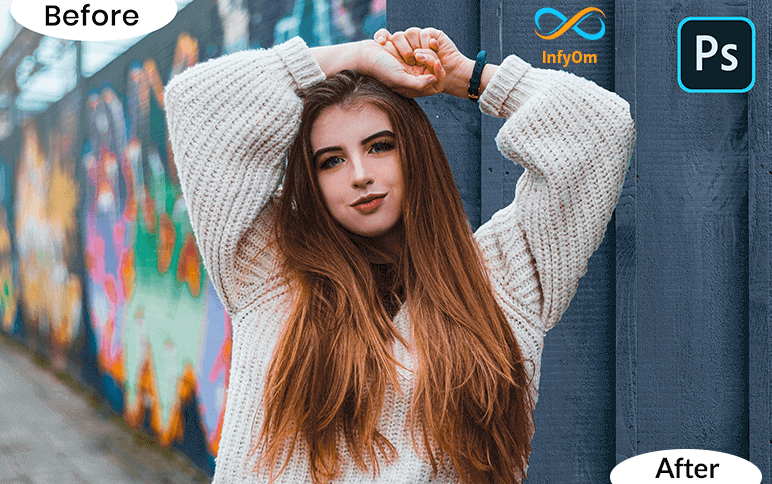
How To Change Background in Photoshop
Any type of user image how to change texture background in this blog learning
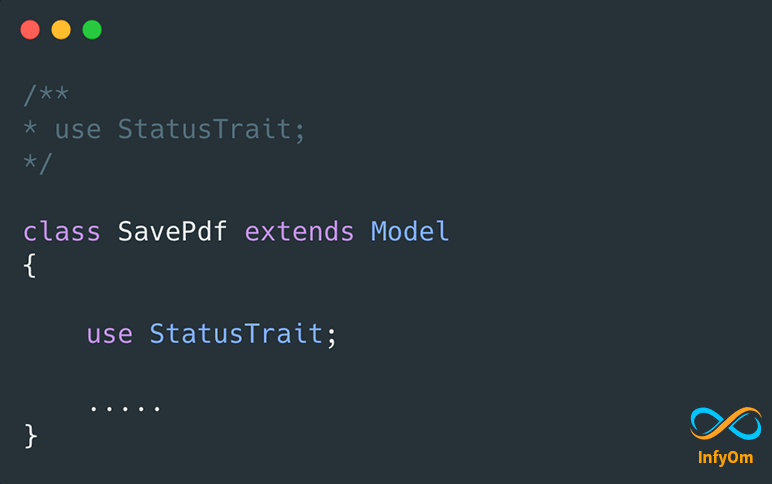
Using Common StatusTrait in Laravel in multiple models
How to create a common Status Trait for the status field for different statuses of models in…
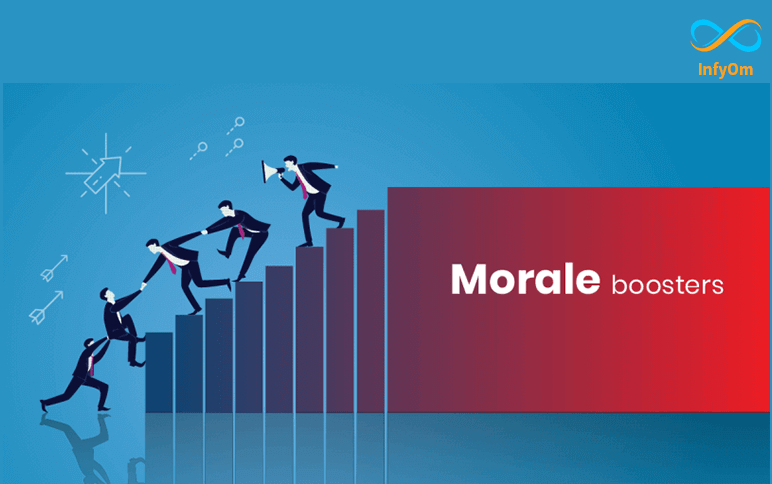
Employee Morale: Play significant role in Business Development
It’s true employee is play significant role in company. The employee is important to organization since it directly…
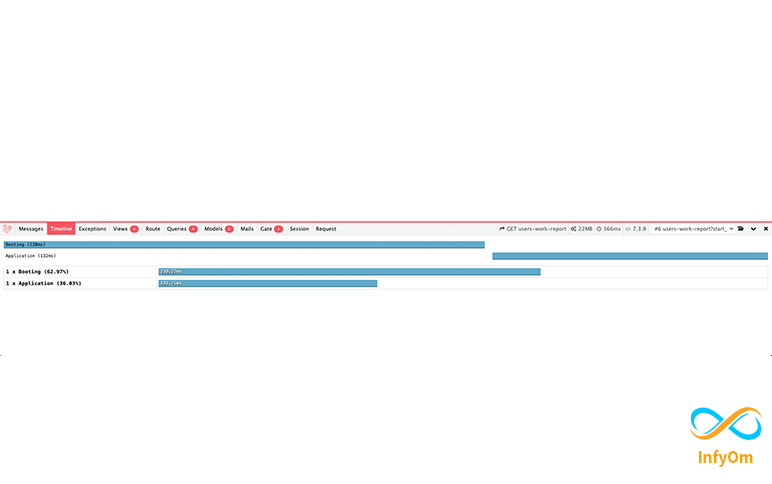
Use Laravel Debugbar’s measure function to optimize application performance
How to use the measure function of Laravel Debugbar to calculate the total execution time of function in…