Recently, I’ve started working on one project where we follow modules patterns and for the same, we have different assets folders for the different modules and the folder named common for assets which are common across all the modules.
So our public folder looks like the following,
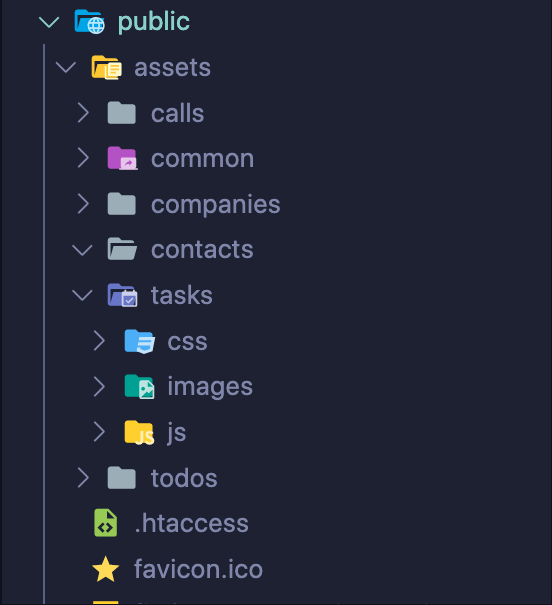
The problem that I started facing was everywhere I needed to give a full path to import/include any of the files from any of the folders. For e.g.
 }})
Even if we have some folder in images to group similar images then it was even becoming longer. For e.g.
 }})
The workaround that I used is, I created one file called helpers.php and created dedicated asset functions for each of the modules. For e.g., for tasks,
if (!function_exists('tasks_asset')) {
/**
* Generate an asset path for the tasks module folder.
*
* @param string $path
* @param bool|null $secure
* @return string
*/
function tasks_asset($path, $secure = null){
$path = "assets/tasks/".$path;
return app('url')->asset($path, $secure);
}
}
With this function, I can use,
 }})
Other advantages it gives are,
- if in future if the path of tasks folder changed, then I do not need to go and update every single import/include.
- I (or any new developer) do not need to remember the long paths and can always use direct function names for modules.
Even I like this pattern so much, so I went further and created dedicated image function as well,
if (!function_exists('tasks_image')) {
/**
* Generate an asset path for the tasks module images folder.
*
* @param string $path
* @param bool|null $secure
* @return string
*/
function tasks_image($path, $secure = null){
$path = "images/".$path;
return tasks_asset($path, $secure);
}
}
So I can use it as,
 }})
Simple and handy functions to use everywhere.