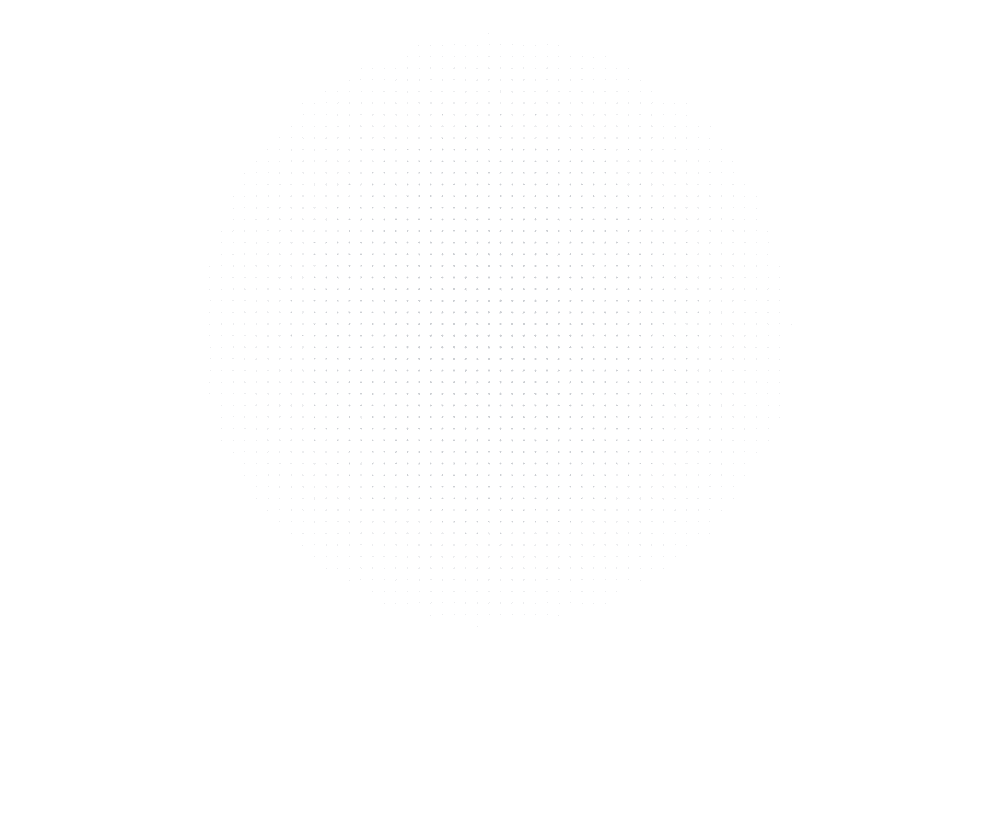
Latest Insights
Explore our blog for the latest trends, tips, tutorials and technologies that are shaping the future of software development.
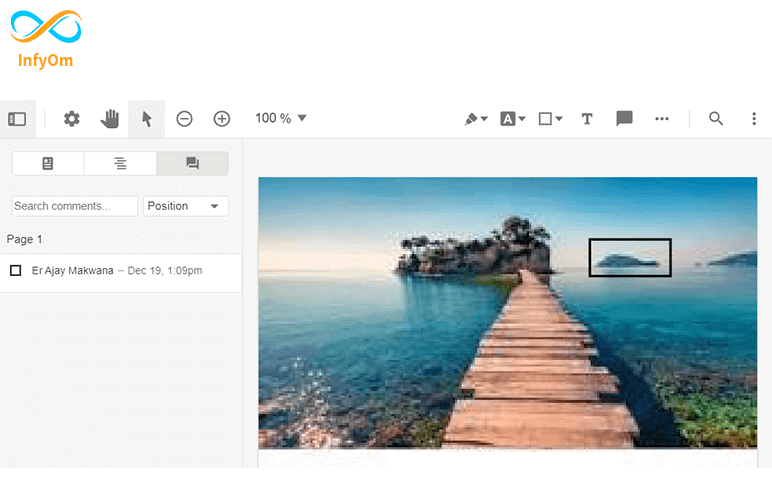
Export annotations of PDFTron using jquery
Export annotation from PDF file using jquery drawn using PDFTron
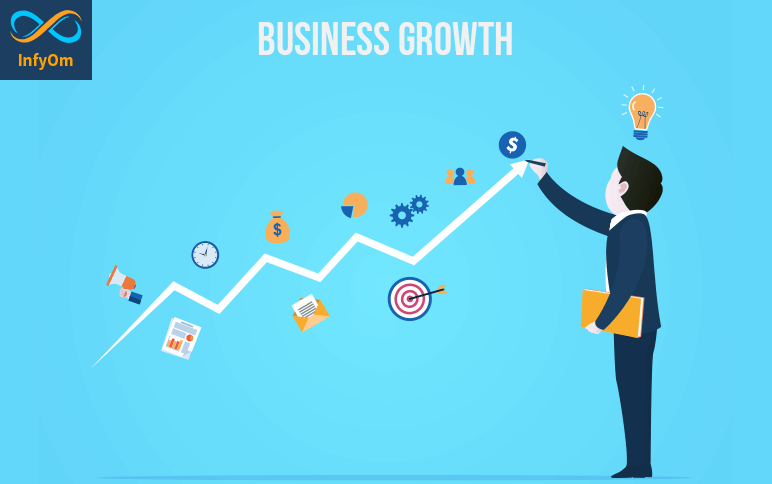
How to increase profit in our business – 2
profit in our business ,costs, sales, price, increase sales, sales Channel
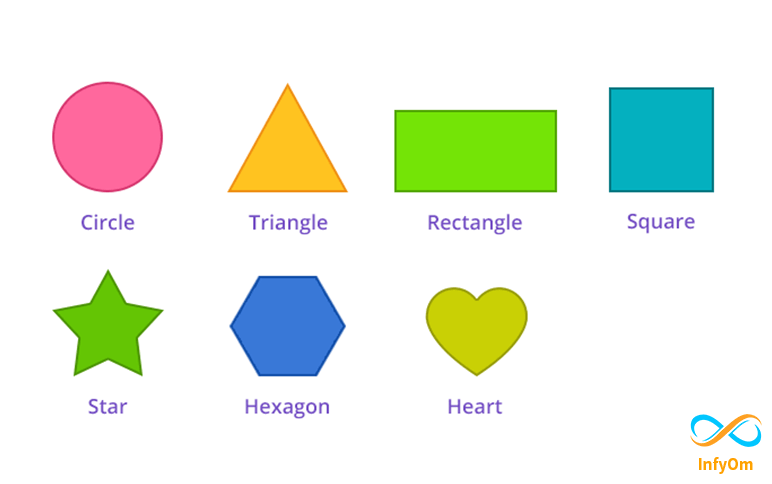
How to create different type shape in android java
capsule shape,round shape,rectangle shape,square shape
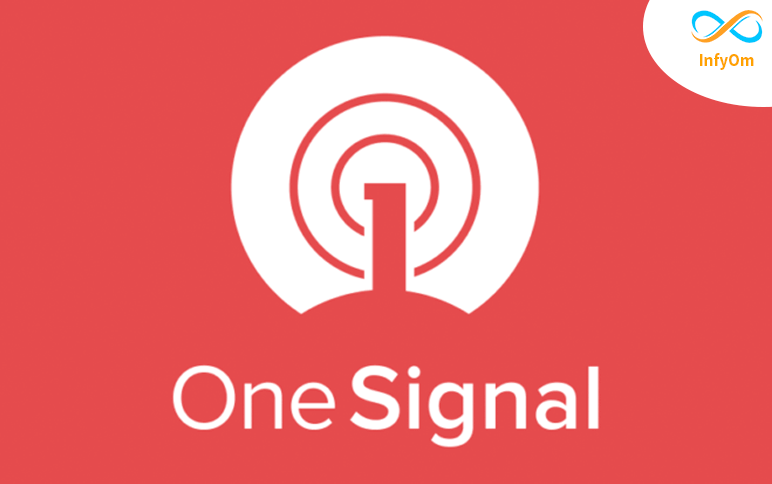
How To Integrate onesignal push notification in Android
When you pick up your phone, the first messages you see push notifications maybe there’s a breaking news alert, game…
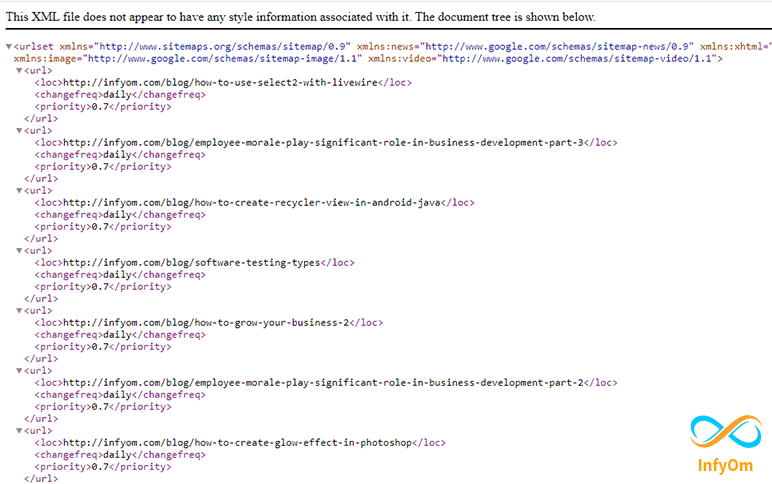
How create a sitemap for your Gatsby site
How create a sitemap for your Gatsby site in react
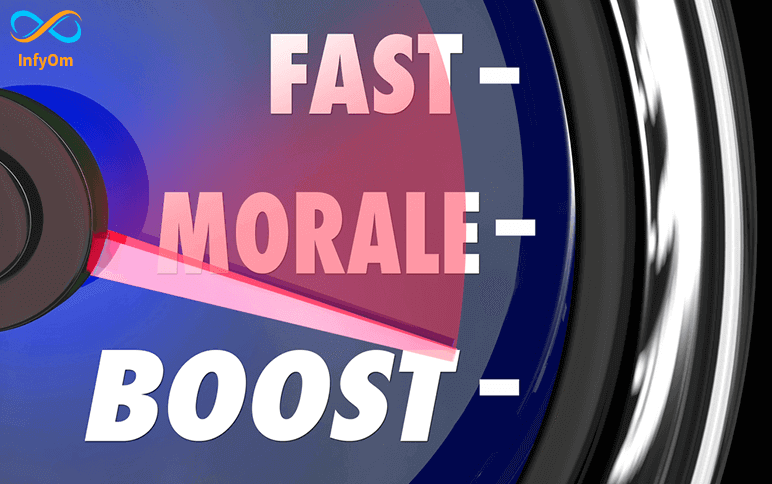
Employee Morale: Play significant role in Business Development Part-3
It’s not possible to measure the Morale directly but we need to look at the various organizational…
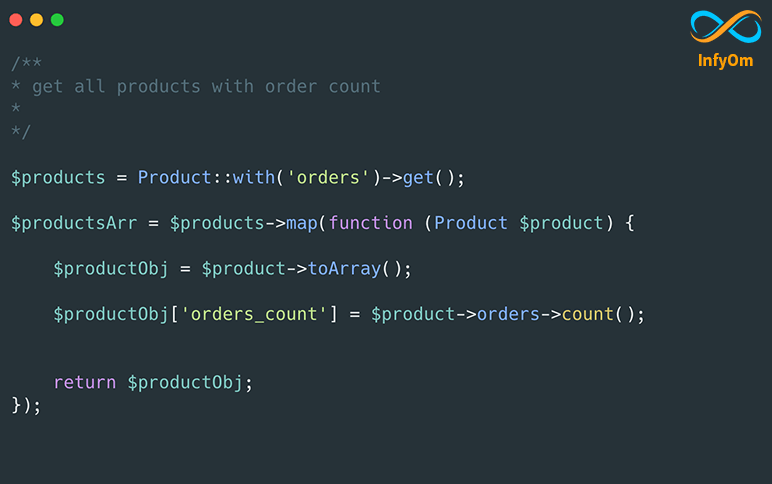
Retrieve count of nested relationship data in Laravel
How to retrieve the count of relationship and nested data in laravel in an optimized…
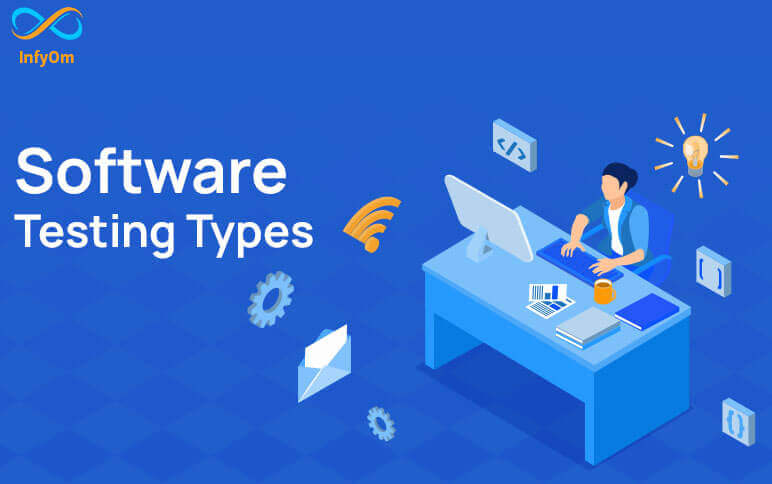
Software Testing Types
The testing is important since it discovers defects/bugs before the delivery to the client, which guarantees the…
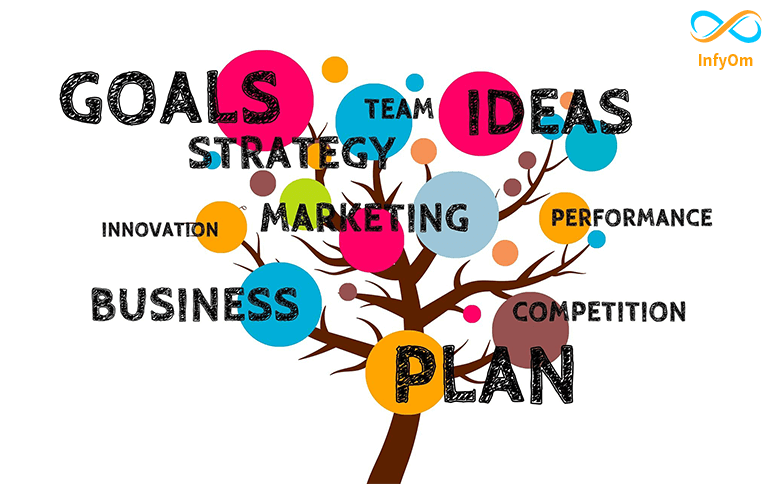
How To Grow Your Business – 2
How to grow you business with develop products and services that are very…