Use of Required Without Validation Rule in Laravel
Laravel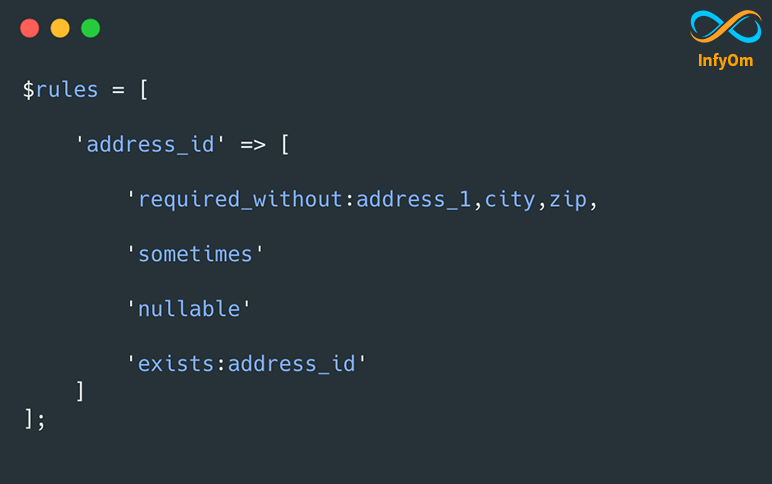
Last month, I got consulting for one Laravel project where we have to perform some complex validations.
The scenario was while creating an order, either the customer can select the existing address from the dropdown or he may have an option to create a new address with all address fields.
And when a customer hits enter, the backend needs to validate, if address_id
is sent into request then it needs to check if that address id exists and then use that address_id for that particular order. Otherwise, it needs to check if required fields (address1, city, zip, country) for the address are sent, then use them, create a new address and use that new address_id
.
so far how validation was happening was manual, so in controller this all manual validation was happening. But I don't find that a proper way. The goal was to do validation from CreateOrderRequest only. so it goes back with proper laravel error messages from a request only and displays them on the page. so we actually do not need to make any manual efforts to make this happen.
That's where required_without
validation rule helped us.
The UI was something like this,
In the above UI, customers can either type Address1, Address2, City and Zip or just go and select an existing address from the dropdown.
To do this validation from form request, we used the required_without
rule as following, 'address_id' => 'required_without:address_1,city,zip|sometimes|nullable|exists:addresses,id',
Now let's try to understand what's happening here. To understand it better let's divide the rules
required_without:address_1,city,zip
sometimes
nullable
exist:addresses,id
1. required_without:address _1,city,zip
This rule validates that address_id
field is required without the presence of address_1
, city
and zip
fields
2. sometimes
This means, address_id
fields will be passed only sometimes and not required all the time. We need this because when address_1
, city
and zip
fields will be present then we do not need it at all.
3. nullable
This means, address_id
fields can be null since it will be null when a customer does not select the address from the dropdown.
4. exist:addresses,id
The passed value in address_id
fields, must exist in the addresses
table.
So this is how we solved this complex validation in a very easy way by using multiple powerful laravel validation rules.
Hope this can help others as well.