Pusher Posts
Send real time notification with Pusher using Laravel and JavascriptLaravel
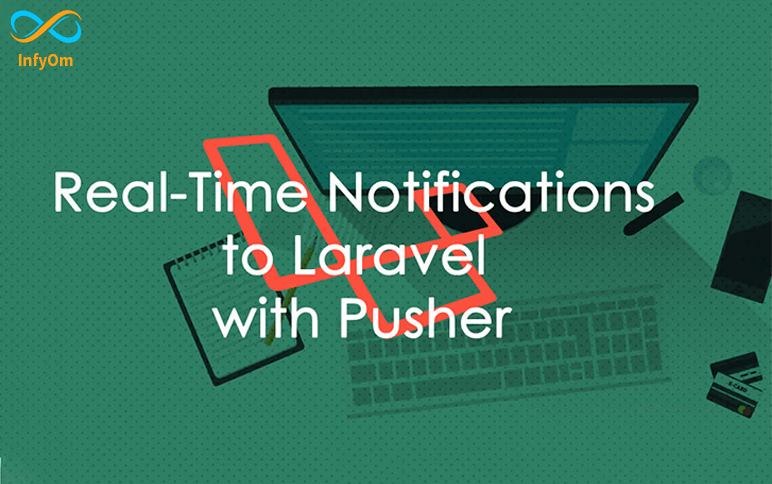
Send real time notification with Pusher using Laravel and JavascriptLaravel
Here we will learn how to send real-time notifications using Pusher + Laravel.
First of all, you need to create an account in the Pusher and get API keys from there.
Setting up your Laravel application
Now we need to install Pusher SDK, you can install it by the composer using the below command,
composer require pusher/pusher-php-server
After the composer is done, we will need to configure Laravel to use Pusher as its broadcast driver, update the below variables in the .env file,
PUSHER_APP_ID=123456
BROADCAST_DRIVER=pusher
// Get the API Keys from your pusher dashboard
PUSHER_APP_ID=XXXXX
PUSHER_APP_KEY=XXXXXXX
PUSHER_APP_SECRET=XXXXXXX
Open config/app.phpand uncomment the "App\Providers\BroadcastServiceProvider::class".
Now we need an event that will be broadcast to the pusher driver. Let's create a NotificationEvent.
php artisan make:event NotificationEvent
This command will create a below file
namespace App\Events;
use Illuminate\Queue\SerializesModels;
use Illuminate\Foundation\Events\Dispatchable;
use Illuminate\Broadcasting\InteractsWithSockets;
use Illuminate\Contracts\Broadcasting\ShouldBroadcast;
class NotificationEvent implements ShouldBroadcast
{
use Dispatchable, InteractsWithSockets, SerializesModels;
public $username;
public $message;
public function __construct($username)
{
$this->username = $username;
$this->message = "{$username} send you a notification";
}
public function broadcastOn()
{
//it is a broadcasting channel you need to add this route in channels.php file
return ['notification-send'];
}
}
Add broadcasting route in channels.php file
Broadcast::channel('notification-send', function ($user) {
return true;
});
Cache Event at Javascript Side
// Initiate the Pusher JS library
var pusher = new Pusher('YOUR_API_KEY', {
encrypted: true
});
// Subscribe to the channel we used in our Laravel Event
var channel = pusher.subscribe('notification-send');
channel.bind('App\\Events\\NotificationEvent', function(data) {
// this is called when the event notification is received...
});
Testing and Setup
Using the below route we can send a notification.
Route::get('test', function () {
event(new App\Events\NotificationEvent('Monika'));
return "Event has been sent!";
});