Package Integration Posts
How to Implement Browser Push Notification in LaravelLaravel
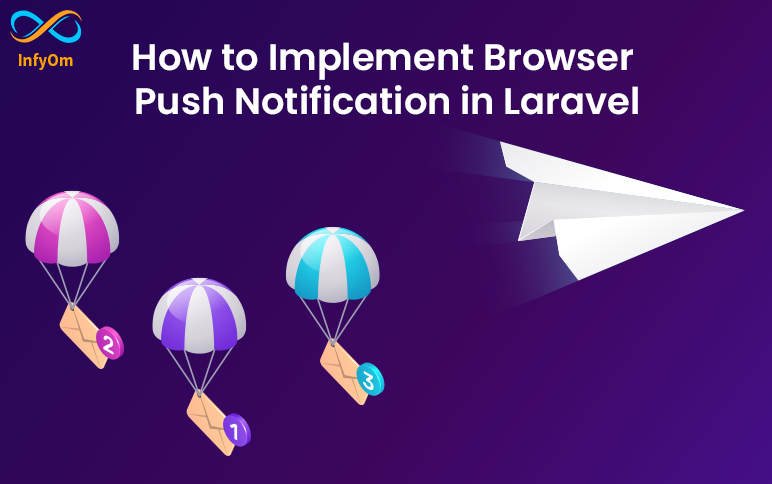
How to Implement Browser Push Notification in LaravelLaravel
You can watch the following tutorial and you can continue reading this article.
Follow the Steps given here for setup push notification.
Step 1: You can quickly install Push via npm
npm install push.js --save
Step 2: Update webpack.mix.js
Add following code into webpack.mix.js
for copy and publish assets like js in the public directory. you can see the example here
mix.copy('node_modules/push.js/bin/push.min.js',
'public/assets/js/push.min.js');
I hope you know how to use laravel mix. you can watch this video tutorial if you want to know more about the laravel mix.
fire, npm run dev
command and publish js.
Step 3: Add assets in blade file
Add script before closing body tag.
<script src="{{ asset('assets/js/push.min.js') }}"></script>
Step 4: Add this code where you want to show a push
// add logo in public dir and use it here
const iconPath = '{{ asset('logo.PNG') }}
Push.create("Hello Shailesh!",{
body: "Welcome to the Dashboard.",
timeout: 5000,
icon: iconPath
});
How to add project as a library to Android StudioAndroid Development
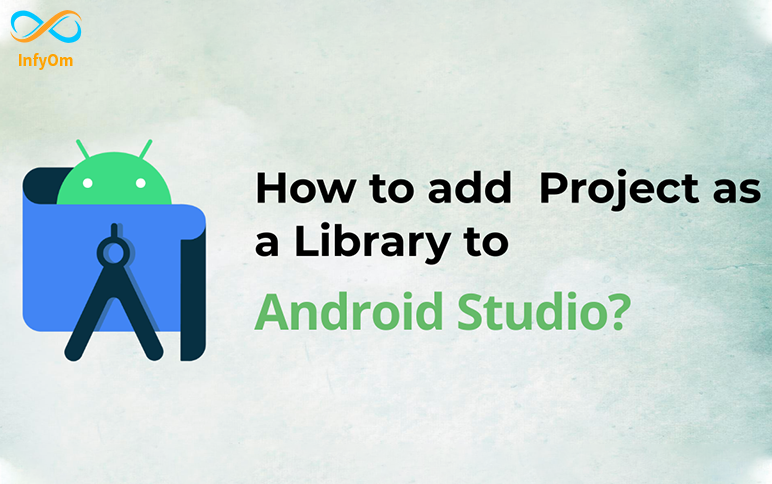
How to add project as a library to Android StudioAndroid Development
But if you want to add another project as a library in your application. For that, you need to add that library project to your application as a module dependency.
1. Open Your Project in Android Studio.
2. You will find the two main directory name samples and others.
3. Go to Android Studio and navigate to File -> New -> Import Module -> Select the library path -> Finish.
4. Import-Module from Directory.
5. Then right-click on the App directory -> Open Module Settings -> Dependencies -> Click on + button inside Declared Dependencies -> Module Dependency -> Select your library -> Ok.
Another way to add Module Dependency
- Open
build.gradle
Of the main module
dependencies {
implementation project(":sample")
}
There can be a situation when we need to modify the library code according to our requirement then in that case we can follow this method.
How to generate User Device API using Laravel One SignalLaravel
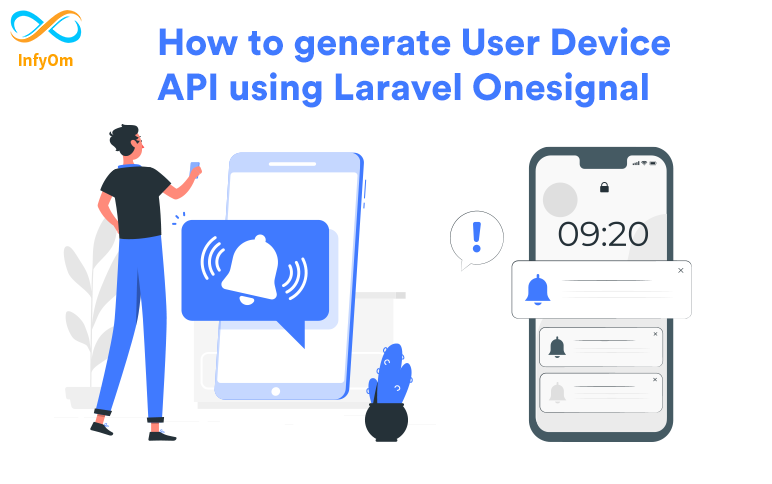
How to generate User Device API using Laravel One SignalLaravel
Generally, we are using a Laravel One Signal package for push notification. if you are planning to use one signal in the mobile application then this package right for you.
Recently, I add a new feature UserDevice. let me explain why I added support for user Device APIs.
We need to create an API to register a device because One Signal sends a push notification using os player id. so, we need to store os_player_id in the backend from the mobile application. So, need to create an API for it.
Now. you can save your time using this package. you can Generate APIs using one artisan command,
php artisan one-signal.userDevice:publish
This command generates the following files,
- UserDeviceAPIController
- UserDeviceAPIRepository
- UserDevice (model)
- Migration So, everything is ready in minutes and delivered an API on the spot.
Also, do not forget to add the following routes to the api.php file.
use App\Http\Controllers\API\UserDeviceAPIController;
Route::post('user-device/register', [UserDeviceAPIController::class, 'registerDevice']);
Route::get('user-device/{playerId}/update-status', [UserDeviceAPIController::class, 'updateNotificationStatus'])
Stisla Templates with JQuery DatatablesLaravel
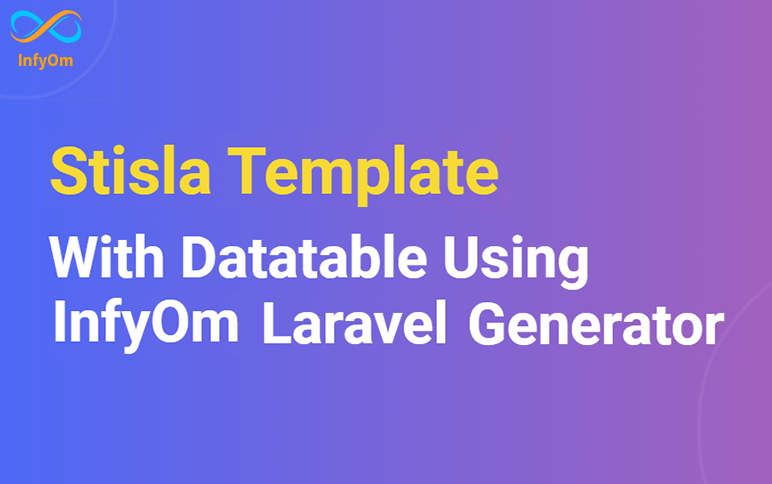
Stisla Templates with JQuery DatatablesLaravel
Today we are going to see how we can generate a data table with one of the most popular a stisla theme.
We can actually do that in minutes with the package that we Recently developed called stisla-templates .
Our team made a great effort into this package and developed it with a new feature. This template package has Jquery Datatable support. So, anyone can easily generate CRUD(scaffold) with a Data table.
Let's see step by step, how we can do that.
You can watch the following video tutorial or follow the article.
Install Packages
Follow the installation steps given in our official documentation of Laravel InfyOm generator and stisla-templates if not installed.
Now, you have to perform the following steps.
composer require yajra/laravel-datatables-oracle:"~9.0"
This package handles the query and frontend stuff.
Register provider and facade on your config/app.php
file.
Now clear your cache and regenerate it using the following command,
php artisan config:cache
We are done with installation and configuration.
Use Generate Scaffold with Datatable
Now I am going to add an option jqueryDT
, at last, to use JQuery Datatables while generating scaffolds. the command looks like
php artisan infyom:scaffold Post --jqueryDT
Enter all required inputs and generate a scaffold of Post.
All views are created inside the posts directory in the resource. Also, the post.js
file is created inside the js directory in assets that are located inside the resource.
Fire the following command for compile and publish the post.js
npm run dev
Now, the data table is ready for use. you can watch the video tutorial here.
How to do payments with stripe checkoutLaravel
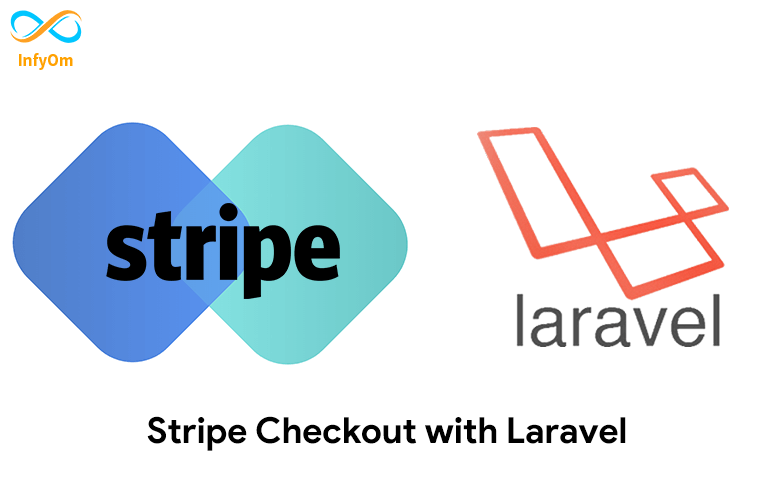
How to do payments with stripe checkoutLaravel
Payments gateways are very useful components of any e-commerce store. One of the popular payment gateways is Stripe. it's becoming more popular nowadays.
Stripe's simple definition is :
We bring together everything that’s required to build websites and apps that accept payments and send payouts globally. Stripe’s products power payments for online and in-person retailers, subscription businesses, software platforms and marketplaces, and everything in between. ~ Stripe
To begin this laravel tutorial, I hope you already have fresh laravel repo.
Stripe Configuration with Laravel
Run the following command to install stripe :
composer require stripe/stripe-php
if you don't have a Stripe account, you'll want to set that up and add your API keys. Add the following to your .env file.
STRIPE_KEY=your-stripe-key
STRIPE_SECRET=your-stripe-secret
Publish Migrations Files From Stripe
php artisan vendor:publish --tag="cashier-migrations"
And Run migrations by hitting the following command
php artisan migrate
Setup Stripe Controller
Now create a stripe controller by hitting the following command:
php artisan make:controller StripeController
namespace App\Http\Controllers;
use Illuminate\Contracts\View\Factory;
use Illuminate\Http\JsonResponse;
use Illuminate\Http\RedirectResponse;
use Illuminate\Http\Request;
use Stripe\Checkout\Session;
use Stripe\Exception\ApiErrorException;
/**
* Class FeaturedCompanySubscriptionController
*/
class StripeControlle extends AppBaseController
{
public function createSession(Request $request)
{
setStripeApiKey();
$session = Session::create([
'payment_method_types' => ['card'],
'customer_email' => $userEmail,
'line_items' => [
[
'price_data' => [
'product_data' => [
'name' => 'Make '.$company->user->first_name.' as featured Company',
],
'unit_amount' => 100 * 100,
'currency' => 'USD',
],
'quantity' => 1,
'description' => '',
],
],
'client_reference_id' => '1234',
'mode' => 'payment',
'success_url' => url('payment-success').'?session_id={CHECKOUT_SESSION_ID}',
'cancel_url' => url('failed-payment?error=payment_cancelled'),
]);
$result = [
'sessionId' => $session['id'],
];
return $this->sendResponse($result, 'Session created successfully.');
}
public function paymentSuccess(Request $request)
{
$sessionId = $request->get('session_id');
//
}
public function handleFailedPayment()
{
//
}
}
Define Routes
Route::post('stripe-charge', 'StripeController@createSession');
Route::get('payment-success', 'StripeController@paymentSuccess');
Route::get('failed-payment', 'StripeController@handleFailedPayment');
Setup From View file
Here we are going to create stripe session from the backend and redirect to the stripe checkout page once we will receive the sessionId from the backend.
Assume that makePaymentURL is something like "APP_URL/stripe-charge".
Now let's say when you hit the submit form of stripe it will call MakePaymentURL and that URL returns your session ID which we will use to redirect to the stripe checkout page.
$(document).on('click', '#makePayment', function () {
$(this).addClass('disabled');
$.post(makePaymentURL, payloadData).done((result) => {
let sessionId = result.data.sessionId;
stripe.redirectToCheckout({
sessionId: sessionId,
}).then(function (result) {
$(this).html('Make Featured').removeClass('disabled');
manageAjaxErrors(result);
});
}).catch(error => {
$(this).html('Make Featured').removeClass('disabled');
manageAjaxErrors(error);
});
});
That's it, after entering proper details into stripe you will get a success callback to a related route, where you can perform related actions.