Laravel Posts
How to Set Application Fee or How to set Destination charges while using Stripe Connect ?Laravel
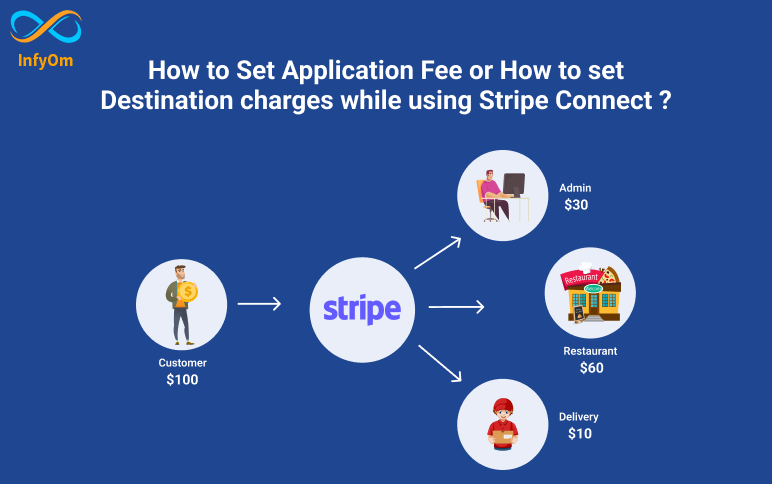
How to Set Application Fee or How to set Destination charges while using Stripe Connect ?Laravel
In our previous blog, we had seen how to integrate stripe connect. Now we are going to see how we can split payments to the application and vendor using stripe checkout.
Let's take a simple example :
The platform is "Shopify", Merchant (Who is selling products on Shopify) & Customer who is going to register with Shopify.
Now When a customer purchases a product with $1000, Now $10 will be considered as an application fee, which is going to transfer to the Platform Account and the rest amount $90 will be transferred to Merchant.
Create Session using Stripe Checkout
public function generationSession($data)
{
$session = \Stripe\Checkout\Session::create([
'payment_method_types' => ['card'],
'customer_email' => $data['email'],
'line_items' => [
[
'name' => "item name here",
'amount' => floatval($data['amount']) * 100,
'currency' => 'usd',
'quantity' => '1',
],
],
'client_reference_id' => $data['reference_id'],
'success_url' => url('payment-success').'?session_id={CHECKOUT_SESSION_ID}',
'cancel_url' => url('failed-payment?error=payment_cancelled'),
'payment_intent_data' => [
'application_fee_amount' => $data['application_fees'] * 100,
'transfer_data' => [
'destination' => $data['user']->stripe_connect_id,
],
],
]);
return $session;
}
It will return the session object return, later you can use the session id to redirect to stripe checkout.
Redirect to checkout using StripeJS
fetch('/generate-sesssion', {
method: 'POST',
})
.then(function(session) {
return stripe.redirectToCheckout({ sessionId: session.id });
});
Check the transaction from your Stripe Dashboard
Once you do the transaction successfully, you can verify whether the application fee is applied or not from your stripe dashboard.
Hope this tutorial helps you.
Integrate CCAvenue Payment Gateway with LaravelLaravel
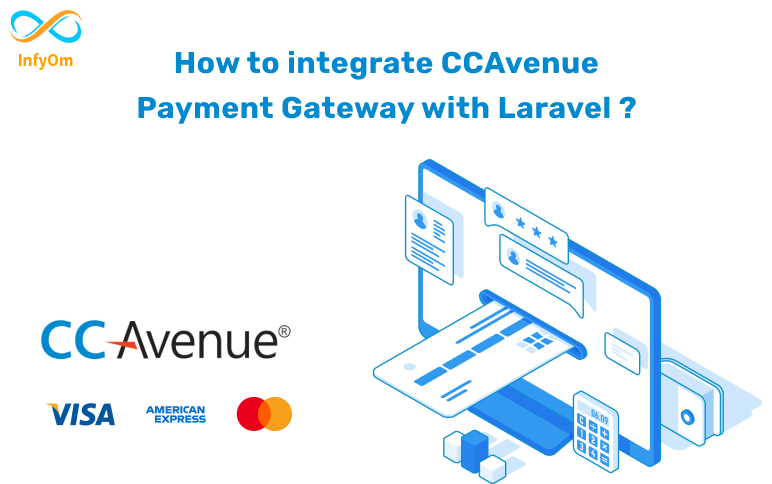
Integrate CCAvenue Payment Gateway with LaravelLaravel
This is the hardest thing as a developer I found, as there is no official documentation available to integrate the CCAvenue. Also, you will not find any tutorial or blog from which you can do the implementation.
So, in this tutorial, we are going to cover how we can integrate the CCAvenue payment gateway with Laravel.
web.php
Route::post('purchase', [CCAvenueController::class, purchaseSubscription']);
CCAvenueController.php
public function purchaseSubscription(Request $request)
{
$input = $request->all();
$input['amount'] = $data['grandTotal'];
$input['order_id'] = "123XSDDD456";
$input['currency'] = "INR";
$input['redirect_url'] = route('cc-response');
$input['cancel_url'] = route('cc-response');
$input['language'] = "EN";
$input['merchant_id'] = "your-merchant-id";
$merchant_data = "";
$working_key = config('cc-avenue.working_key'); //Shared by CCAVENUES
$access_code = config('cc-avenue.access_code'); //Shared by CCAVENUES
$input['merchant_param1'] = "some-custom-inputs"; // optional parameter
$input['merchant_param2'] = "some-custom-inputs"; // optional parameter
$input['merchant_param3'] = "some-custom-inputs"; // optional parameter
$input['merchant_param4'] = "some-custom-inputs"; // optional parameter
$input['merchant_param5'] = "some-custom-inputs"; // optional parameter
foreach ($input as $key => $value) {
$merchant_data .= $key . '=' . $value . '&';
}
$encrypted_data = $this->encryptCC($merchant_data, $working_key);
$url = config('cc-avenue.url') . '/transaction/transaction.do?command=initiateTransaction&encRequest=' . $encrypted_data . '&access_code=' . $access_code;
return redirect($url);
}
Manage Callback
public function ccResponse(Request $request)
{
try {
$workingKey = config('cc-avenue.working_key'); //Working Key should be provided here.
$encResponse = $_POST["encResp"];
$rcvdString = $this->decryptCC($encResponse, $workingKey); //Crypto Decryption used as per the specified working key.
$order_status = "";
$decryptValues = explode('&', $rcvdString);
$dataSize = sizeof($decryptValues);
}
Other Encryption functions
public function encryptCC($plainText, $key)
{
$key = $this->hextobin(md5($key));
$initVector = pack("C*", 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09, 0x0a, 0x0b, 0x0c, 0x0d, 0x0e, 0x0f);
$openMode = openssl_encrypt($plainText, 'AES-128-CBC', $key, OPENSSL_RAW_DATA, $initVector);
$encryptedText = bin2hex($openMode);
return $encryptedText;
}
public function decryptCC($encryptedText, $key)
{
$key = $this->hextobin(md5($key));
$initVector = pack("C*", 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09, 0x0a, 0x0b, 0x0c, 0x0d, 0x0e, 0x0f);
$encryptedText = $this->hextobin($encryptedText);
$decryptedText = openssl_decrypt($encryptedText, 'AES-128-CBC', $key, OPENSSL_RAW_DATA, $initVector);
return $decryptedText;
}
public function pkcs5_padCC($plainText, $blockSize)
{
$pad = $blockSize - (strlen($plainText) % $blockSize);
return $plainText . str_repeat(chr($pad), $pad);
}
public function hextobin($hexString)
{
$length = strlen($hexString);
$binString = "";
$count = 0;
while ($count < $length) {
$subString = substr($hexString, $count, 2);
$packedString = pack("H*", $subString);
if ($count == 0) {
$binString = $packedString;
} else {
$binString .= $packedString;
}
$count += 2;
}
return $binString;
}
That's it.
So that is how you can integrate the CCAvenue Payment Gateway with Laravel.
How to create artisan command in Laravel with arguments supportLaravel
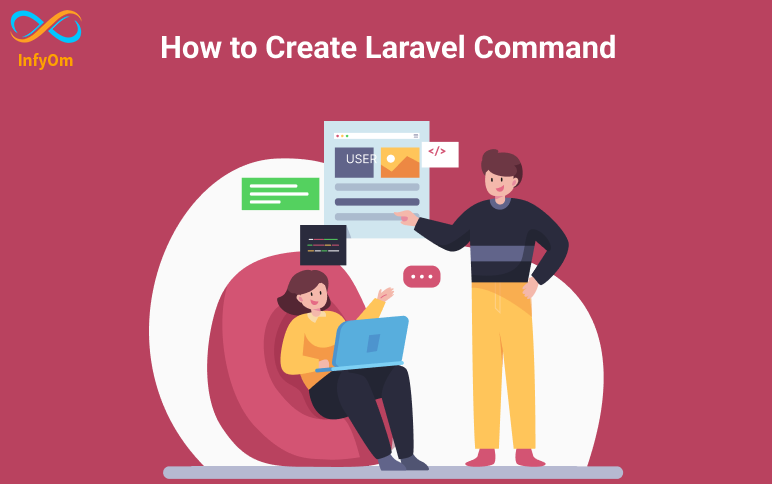
How to create artisan command in Laravel with arguments supportLaravel
Create your own Command
In Laravel you can write your own customized command. Generally, commands will be stored in app\Console\Commands
.
Generate Command
You can generate a command using the default Laravel artisan command :
php artisan make:command AppointmentReminder
Understanding Command
There is main 2 parts in the console command.
- Signature property
- Handle() method
$signature is the command name where you can specify the command name. if you are giving the name "send:reminder" then you have to fire the full command as follows.
php artisan send:reminder
Defining Arguments
You can define optional and required arguments into curly braces as follow.
protected $signature = 'send:reminder {appointmentID}';
// For the Optional argument you have to define ? in last
'mail:send {appointmentID?}'
Fetching Arguments
After executing the command if the argument is passed you have to fetch it. for that, you can use the default function as follow.
public function handle(): void
{
$appointmentID = $this->argument('appointmeentID');
}
You can use Options in the same manner as we did for the arguments.
Executing Commands from code
Route::post('/send-reminders', function (string $user) {
Artisan::call('send:reminder', [
'appointmentID' => 123
]);
// ...
});
Laravel Jobs - How Jobs works in Laravel?Laravel
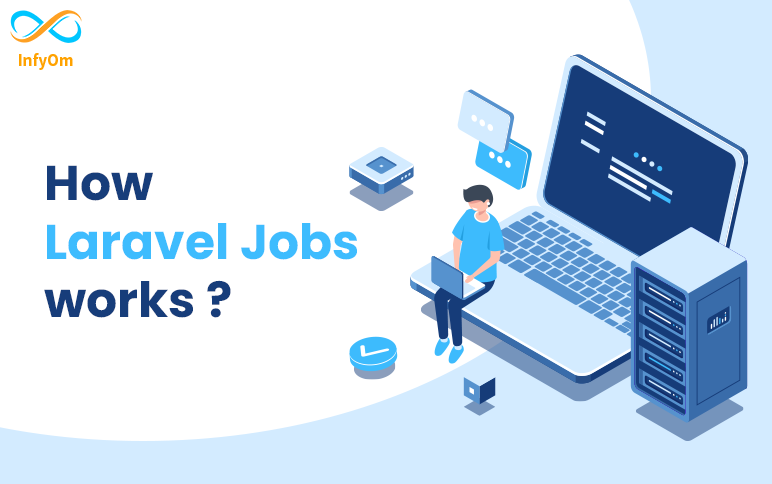
Laravel Jobs - How Jobs works in Laravel?Laravel
Out of 100 only 30/40 people know about Laravel jobs and how its works or where we can use it.
When there is a need to use it specifically or the senior team asked that "You have to use Laravel Jobs", generally then people come to the web and search :
- "How Laravel Jobs works ?" Or "Laravel Jobs works in Local Environment?"
In this tutorial, we are going to understand the basic functionality of jobs and their usage in simple words without any complex examples.
What is a Job?
Jobs generally contain a portion of code that runs in the background without blocking any other code.
You first need to create a job and you can write a portion of code into it.
Then you have to dispatch that job.
How Job works?
Let's say we want to send emails to 100 users. In general, terms what we do is: Execute a loop over each user and send emails one by one.
That will definitely take time and we also have to wait for the response.
Overcome that issue by using jobs.
First, we need to create a job that accepts 10 users and sends emails.
Now we will execute a loop over 100 users, so basically we have to dispatch the job 10 times.
So basically 10 times jobs will be dispatched and immediately we will get a response, as jobs run in the background without waiting for any response.
Hope its more clear now.
How to implement Paystack payment gateway in laravel applicationLaravel
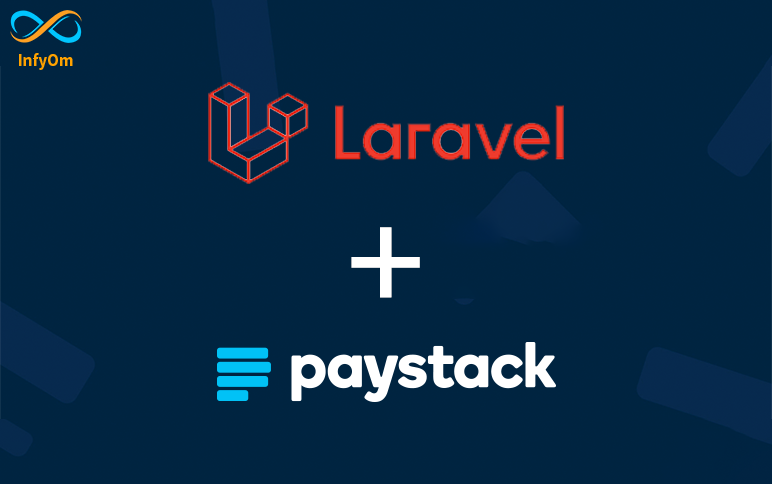
How to implement Paystack payment gateway in laravel applicationLaravel
Paystack is the most popular payment gateway in Africa. Paystack has supported ZAR currencies.
It’s very simple to implement in your laravel application
Install package
composer require unicodeveloper/laravel-paystack
Once Laravel Paystack is installed, you need to register the service provider. Open up config/app.php and add the following to the providers key.
'providers' => [
...
Unicodeveloper\Paystack\PaystackServiceProvider::class,
...
]
Register the Facade
'aliases' => [
...
'Paystack' =>
Unicodeveloper\Paystack\Facades\Paystack::class,
...
]
Note: Make sure you have /payment/callback registered in Paystack Dashboard https://dashboard.paystack.co/#/settings/developer
Set Paystack credentials into config/paystack.php
return [
/**
* Public Key From Paystack Dashboard
*/
'publicKey' => 'PAYSTACK KEY',
/**
* Secret Key From Paystack Dashboard
*/
'secretKey' => 'PAYSTACK SECRET KEY',
/**
* Paystack Payment URL
*/
'paymentUrl' => 'https://api.paystack.co',
/**
* Optional email address of the merchant
*/
'merchantEmail' => 'EMAIL ADDRESS',
];
Configure the route. Enter the code in your web.php file in the route directory
//Paystack Route
Route::get('paystack-onboard', [PaystackController::class, 'redirectToGateway'])->name('paystack.init');
Route::get('paystack-payment-success',
[PaystackController::class, 'handleGatewayCallback'])->name('paystack.success');
Then after create one controller PaystackController.php
public function redirectToGateway(Request $request)
{
try{
$request->request->add([
"email" => "Your email address",
"orderID" => "123456", // anything
"amount" => 150 * 100,
"quantity" => 1, "currency" => "ZAR", // change as per need
"reference" => Paystack::genTranxRef(),
"metadata" => json_encode(['key_name' => 'value']), // this should be related data
]);
return Paystack::getAuthorizationUrl()->redirectNow();
}catch(\Exception $e) {
return Redirect::back()->withMessage(['msg'=>'The paystack token has expired. Please refresh the page and try again.', 'type'=>'error']);
}
}
public function handleGatewayCallback(Request $request)
{
$paymentDetails = Paystack::getPaymentData();
dd($paymentDetails);
}
That's it. Enjoy.
A look at what coming to Laravel 10Laravel
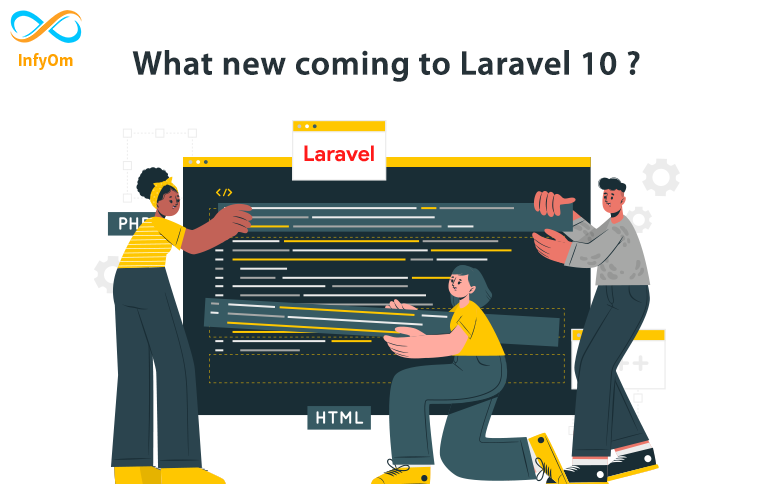
A look at what coming to Laravel 10Laravel
I am personally really excited about Laravel 10, are you also excited :) ?. the expected release date for Laravel 10 is 7th February 2023.
In this tutorial, we are going to cover new features and changes from Laravel 10.
Release Date
Laravel 10 will be released by 7th February 2023 and below is a list of annual release dates.
- Laravel 9: February 8th, 2022
- Laravel 10: February 7th, 2023
- Laravel 11: February 6th, 2024
Drop Support for PHP 8.0
Laravel 10 dropping support for PHP <= 8.0, the minimum requirement to run Laravel 10 is PHP 8.1
Deprecations from Laravel 9
In Laravel 10 some of Laravel 9 methods will be deprecated, here is the list.
- Remove various deprecations
- Remove deprecated dates property
- Remove handleDeprecation method
- Remove deprecated assertTimesSent method
- Remove deprecated ScheduleListCommand's $defaultName property
- Remove deprecated Route::home method
- Remove deprecated dispatchNow functionality
Native type declarations in Laravel 10 skeleton
In Laravel 10 the code userland generated by framework will contain the type-hints and return types.
Types will be added into latest PHP type-hinting features to Laravel projects without breaking backward compatibility at the framework level:
- Return types
- Method arguments
- Redundant annotations are removed where possible
- Allow user land types in closure arguments
- Does not include typed properties
Any Many more
Hope that will be usefull.
Some Laravel tips that we must need to knowLaravel
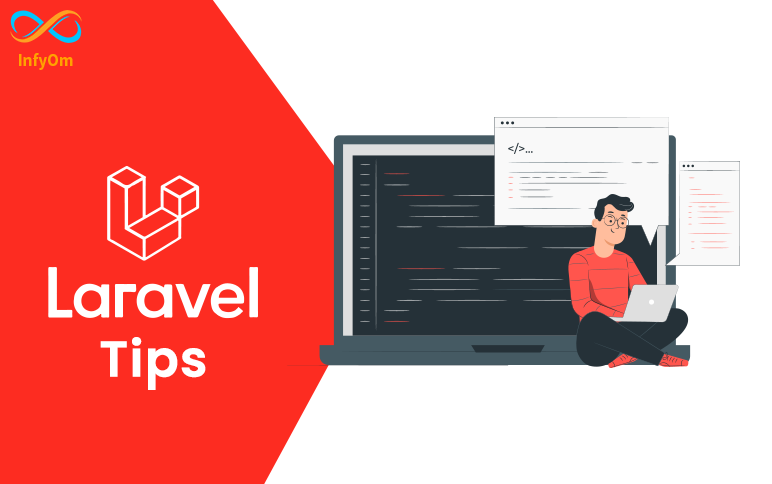
Some Laravel tips that we must need to knowLaravel
1) Null safe operator
From PHP 8 you can use Null Safe Operator
How we are doing null checking code in PHP < 8.0
$country = null;
if ($session !== null) {
$user = $session->user;
if ($user !== null) {
$address = $user->getAddress();
if ($address !== null) {
$country = $address->country;
}
}
}
PHP 8 allows you to write this:
$country = $session?->user?->getAddress()?->country;
2) Ternary condition
We have used this ternary condition for checking null value
isset($user->image) ? $user->image : null
We can short above condition like this
$user->image ?? null
3) Clone a model
You can clone a model using replicate(). It will create a copy of the model into a new, non-existing instance.
$user = App\User::find(1);
$newUser = $user->replicate();
$newUser->save();
4) Default Relationship Models
Laravel provides a handy withDefault() method on the belongsTo relationship that will return a model object even when the relationship doesn't actually exist.
class Post extends Model
{
public function user()
{
return $this->belongsTo(User::class)->withDefault();
}
}
Now, if we try to access the $post->user relationship, we'll still get a User object even when it does exist in the database. This is known as the "null object" pattern and helps eliminate some of those if ($post->user) conditional statements.
For more information read Laravel withDefault() doc.
5) Save models and relationships
You can save a model and its corresponding relationships using the push() method.
class User extends Model
{
public function phone()
{
return $this->hasOne('App\Phone');
}
}
$user = User::first();
$user->name = "Peter";
$user->phone->number = '1234567890';
$user->push(); // This will update both user and phone record in DB
Hope it will be helpful.
Thanks
How to implement Laravel impersonateLaravel
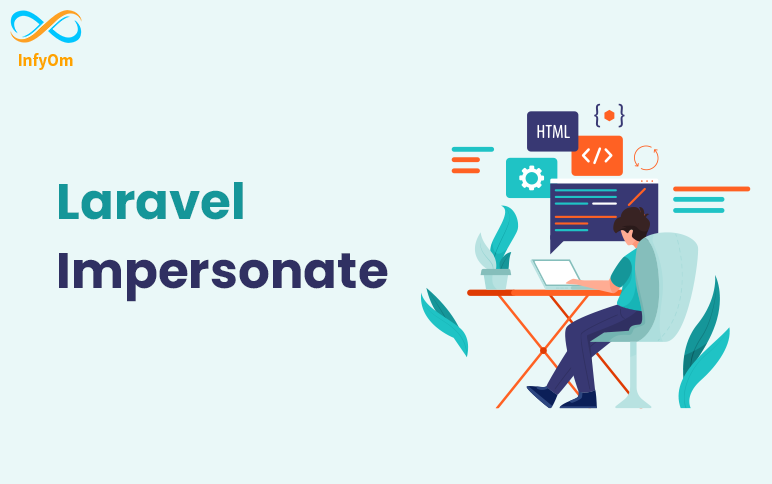
How to implement Laravel impersonateLaravel
What is user impersonate and why we use it in projects?
- User Impersonate is useful when we want to login on behalf of admin user.
- When we are a super-admin and we want to know about our users what they do in their account then this package is useful to check their activity.
- If you implemented impersonate in your project then you need to maintain it because it may cause a security issue as well as user's privacy issue.
Here is a full documentation of laravel impersonate: https://github.com/404labfr/laravel-impersonate
So let's start with installing impersonate on your project copy the below code where we will install this package using composer.
composer require lab404/laravel-impersonate
Add the service provider at the end of your config/app.php
:
'providers' => [
// ...
Lab404\Impersonate\ImpersonateServiceProvider::class,
],
Add the trait Lab404\Impersonate\Models\Impersonate
to your User model.
Using the built-in controller
In your routes file, under web middleware, you must call the impersonate
route macro.
Route::impersonate();
// Where $id is the ID of the user you want impersonate
route('impersonate', $id)
// Or in case of multi guards, you should also add `guardName` (defaults to `web`)
route('impersonate', ['id' => $id, 'guardName' => 'admin'])
// Generate an URL to leave current impersonation
route('impersonate.leave')
Blade
There are three Blade directives available.
When the user can impersonate
@canImpersonate($guard = null)
<a href="{{ route('impersonate', $user->id) }}">Impersonate this user</a>
@endCanImpersonate
When the user can be impersonated
@canBeImpersonated($user, $guard = null)
<a href="{{ route('impersonate', $user->id) }}">Impersonate this user</a>
@endCanBeImpersonated
When the user is impersonated
@impersonating($guard = null)
<a href="{{ route('impersonate.leave') }}">Leave impersonation</a>
@endImpersonating
That's it. Enjoy.