Learnings Posts
Some Laravel tips that we must need to knowLaravel
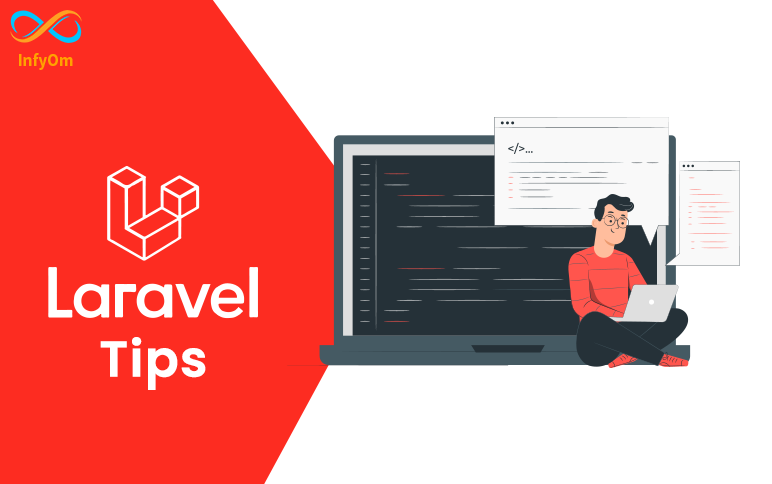
Some Laravel tips that we must need to knowLaravel
1) Null safe operator
From PHP 8 you can use Null Safe Operator
How we are doing null checking code in PHP < 8.0
$country = null;
if ($session !== null) {
$user = $session->user;
if ($user !== null) {
$address = $user->getAddress();
if ($address !== null) {
$country = $address->country;
}
}
}
PHP 8 allows you to write this:
$country = $session?->user?->getAddress()?->country;
2) Ternary condition
We have used this ternary condition for checking null value
isset($user->image) ? $user->image : null
We can short above condition like this
$user->image ?? null
3) Clone a model
You can clone a model using replicate(). It will create a copy of the model into a new, non-existing instance.
$user = App\User::find(1);
$newUser = $user->replicate();
$newUser->save();
4) Default Relationship Models
Laravel provides a handy withDefault() method on the belongsTo relationship that will return a model object even when the relationship doesn't actually exist.
class Post extends Model
{
public function user()
{
return $this->belongsTo(User::class)->withDefault();
}
}
Now, if we try to access the $post->user relationship, we'll still get a User object even when it does exist in the database. This is known as the "null object" pattern and helps eliminate some of those if ($post->user) conditional statements.
For more information read Laravel withDefault() doc.
5) Save models and relationships
You can save a model and its corresponding relationships using the push() method.
class User extends Model
{
public function phone()
{
return $this->hasOne('App\Phone');
}
}
$user = User::first();
$user->name = "Peter";
$user->phone->number = '1234567890';
$user->push(); // This will update both user and phone record in DB
Hope it will be helpful.
Thanks
How to Increase Sales from UpworkSales
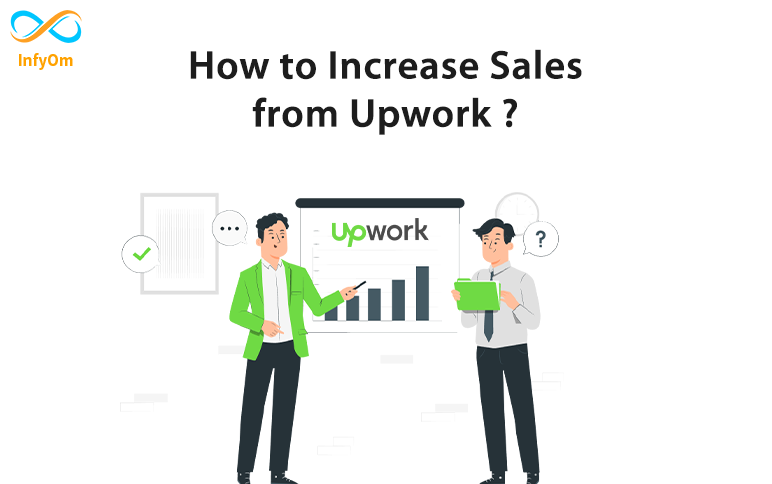
How to Increase Sales from UpworkSales
Upwork is a platform that connects freelancers and clients for a wide range of services including sales. If you're looking to increase your sales on Upwork, here are some tips that can help:
-
Build a strong profile
-
Improve your proposal
-
Be Responsive and Professional
-
Offer added value
-
Use Upwork's tools and resources
Build a strong profile
Your Upwork profile is essentially your online resume. Make sure it accurately reflects your skills, experience, and qualifications. Use a clear, professional profile picture and highlight any relevant certifications or achievements.
Improve your proposal
When you apply for a job on Upwork, you will need to write a proposal explaining why you are the best candidate for the job. Read the job posting carefully and tailor your submission to the client's specific needs. Emphasize your relevant experience and skills and provide examples of your work.
Be Responsive and Professional
Once you've landed a job on Upwork, it's important to communicate effectively with your clients and meet deadlines. Respond to messages promptly and be respectful and professional in your interactions. This will help you build a positive reputation on the platform and increase your chances of getting repeat business or referrals.
Offer added value
In addition to getting the job done to the best of your ability, consider offering added value to your customers. This may be in the form of additional resources or advice or offering to complete additional tasks outside the scope of the original job. This can help you stand out from other freelancers and increase your chances of getting repeat business.
Use Upwork's tools and resources
Upwork offers a variety of tools and resources for freelancers, including the ability to track their time, create invoices, and manage their finances. Be sure to take advantage of these resources to streamline your work and make it easier for customers to do business with you.
Following these tips can increase your sales and build a successful freelance business on Upwork. Good luck!
How to Get Leads from LinkedInSales
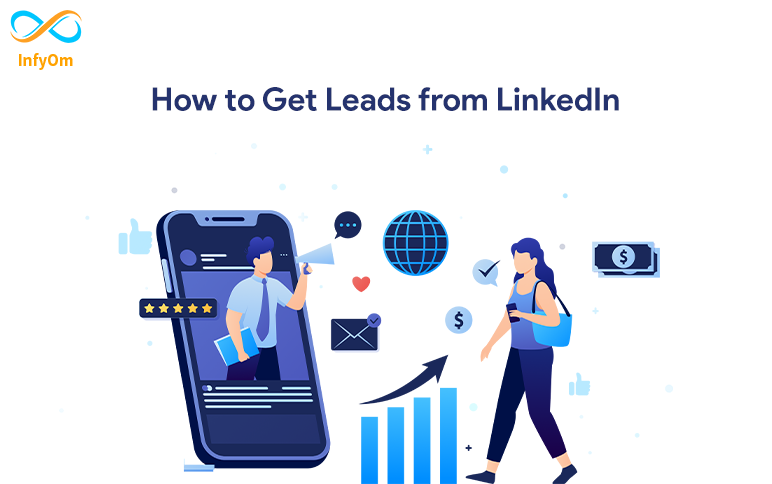
How to Get Leads from LinkedInSales
Add connections to your network
If you spend a minute or more each workday clicking the "Connect" button on the "People You May Know" list that LinkedIn posts in your feed, you'll expand your network, and you'll be known as a network expander. will, which is equally important.
Remember: Everyone you talk to about business or meet during a business day is a potential LinkedIn connection.
Build your lead list
Spend five minutes a day checking your contacts' connections to see who you don't know personally but would like to meet. Note down who you want to introduce. Start with the "recommendations" first, as those are likely the strongest connections of the LinkedIn user you're looking at.
Ask for recommendations outside of your LinkedIn account via email or phone. You will get a quick reply. (And you'll get a chance to quickly reconnect with your connections.)
Follow up with your current customers and prospects
Spend another two minutes each day searching for your current clients and top prospects. Find out if they have a company page. If they do, follow through and monitor.
Join groups
LinkedIn lets you connect with people who are in groups with you. Use this as a targeted way to add value to others, share insights, and build your network with prospects. Invest five minutes in this every day.
Use LinkedIn to celebrate the achievements of others
When you see a news story or post that provides good news about your client or prospect or any key contact, share the news as a status update. Identify a person with an "@" reply. It will ensure that they receive the mentioned notification. Spend a minute a day on this.
Write a recommendation
Securing LinkedIn recommendations is often difficult, if only because it takes time for the author to log in, write, and post.
Instead of waiting for someone to recommend you, take five minutes a day to write and post (reality-based) recommendations for your customers and key contacts. Once your contact approves the text, the recommendation will appear on his/her LinkedIn account.
How to write an email to a potential clientSales
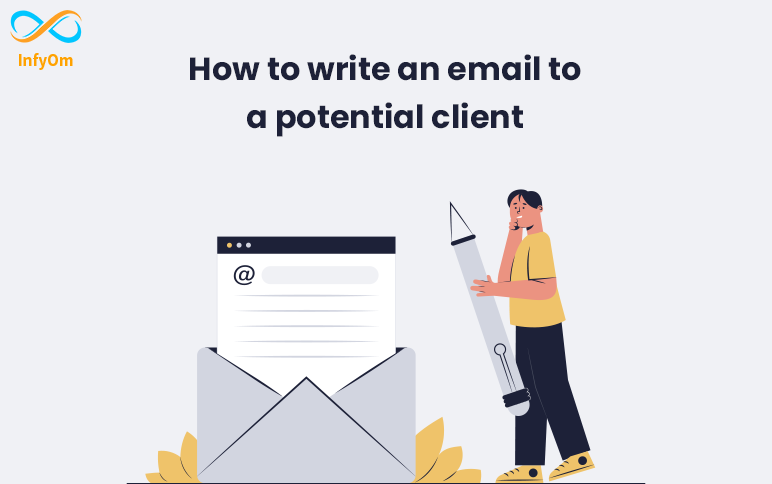
How to write an email to a potential clientSales
Reaching sales prospects over email is an opportunity to sell and develop a working relationship with a new client.
Write a subject line
The first step in writing a strong email to a prospect is to consider the subject line. The first thing a potential client will see in an email is the subject line, so it's important that it persuades the recipient to open the email. Here are some strategies you can use to write a compelling subject line:
Here's what you should do if you want to write good email subject lines:
- Use personalization.
- Ask an engaging question.
- Use concise and action-oriented language.
- Take advantage of scarcity and exclusivity.
Give information about yourself.
You will more likely gain traction if they already know, like, and trust you. But everyone has to start somewhere, right?
If they've never received a communication from you, tell them a little about yourself in a way that feels warm and authentic. You must convey who you are and why they should listen to you. At the same time, it's essential to make it about them. For example, your email sales introduction could look something like this:
"My name is [name], and I'm contacting you because..."
Close the email with a salutation
You can include a closing salutation that matches the level of formality you used to open the email. Here are a few examples of closing salutations for professional emails:
- Thank You
- Best wishes
- Regards
- Looking forward to hearing from you
- Sincerely
Top Laravel packages that you need in 2022Laravel
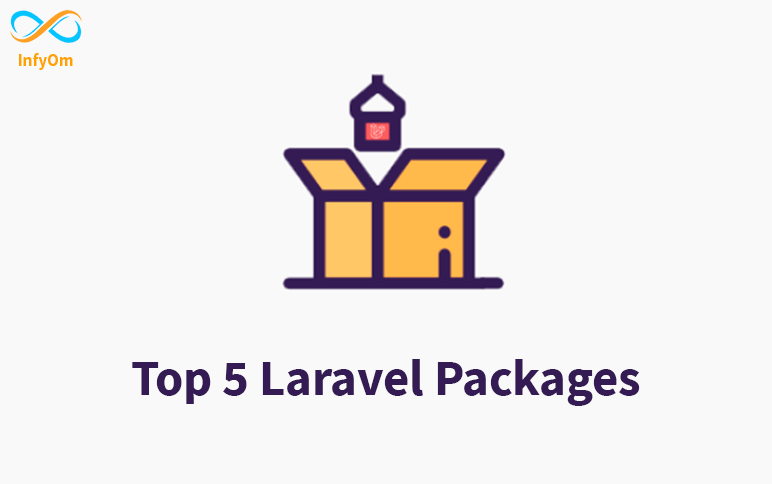
Top Laravel packages that you need in 2022Laravel
What is Laravel?
Laravel is the most popular PHP framework right now to develop web applications, it offers a very easy environment and services for developers.
In this blog, we are going to know about the packages that we must have to use while developing any laravel application.
Best Laravel Packages
Here we are going to see some best and top laravel packages that will help you to optimize your application performance and it's also very useful while doing the development.
IDE Helper
Github: https://github.com/barryvdh/laravel-ide-helper
It's a very helpful package and saves lots of time for the developer.
It will generate the helper file which enables our IDE to provide accurate autocompletion while doing the development.
Laravel Debugbar
Github : https://github.com/barryvdh/laravel-debugbar
This is very helpful when we have to check the page performance, in sense of how many queries are firing on the specific page? , how many models are loading? etc.
We can show the total processing time of the page, and the query results time too. by using that results we can do some refactor to our code and make our application more optimized.
Spatie Medialibrary
Github : https://github.com/spatie/laravel-medialibrary
This package is very useful when we are doing file uploads. also, it allows us to upload files to the s3 (AWS) very easily by changing just the file system driver.
The main functionality it has is it allows us to associate files with the Eloquent models.
Spatie Role Permission
Github : https://github.com/spatie/laravel-permission
It's 2022 and still, lots of developers are using the custom roles/permissions management. they even didn't familiar that this package have capabilities to manage each role/permissions management with a specific Eloquent model too.
We can assign roles or permissions to the user model or even any model. later we can check it via the middleware that this package is providing.
Ziggy
Github : https://github.com/tighten/ziggy
Before using this package you must need to implement the named routes into your laravel application.
Normally people can just provide a hardcoded URL into the JS file while doing the AJAX calls. But with this package, you can use the route we are using in blade files.
This allows us to use the route()
helper method in the JS files.
How to Make Sales and Marketing Meetings More Effective and ImpactfulSales
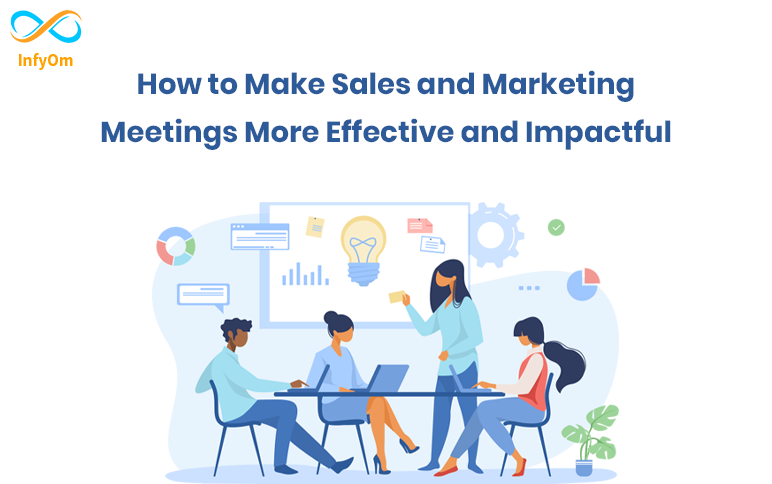
How to Make Sales and Marketing Meetings More Effective and ImpactfulSales
When done effectively, regular sales meetings are crucial to your team's success. In addition to sharing important updates and enabling group discussions, they can also help motivate your sales team.
What was supposed to be a way to make your team more successful turns into another series of updates. Before you know it, your sales representatives are starting to get scared by taking time out of their days to attend.
Establish expectations
When it comes to meetings that involve a lot of people, it is good to set some basic rules in advance. If your sales meetings are going overtime, you might want to consider addressing expectations. To save time and make sure you don't get sidetracked, you can:
- Make sure participants know they should be prepared
- All participants are required to participate
- Control the time spent on discussion topics
Set a goal
The tradition of having a sales meeting once a week is not enough reason to hold a meeting. Yes, you want to block time in your calendar so that your sales team can get together, but it's okay to leave it.
At the end of the day, meetings without a specific purpose seem meaningless and just turn into another calendar entry. What’s worse, they can negatively affect your team’s performance for the rest of the day.
Review the results before the sales meeting
Depending on the size of your team, it may take time to review their results. That’s why your best bet is that they deliver the data before the meeting. The most comprehensive way to do this is to have a live document that they can update in advance.
Make your sales meetings exciting
Just because it’s a sales meeting, doesn’t mean it needs all the data and is no fun. Don’t forget that your team will pick up your energy. In other words, if you treat the meeting as a task, your team will not be more excited than you.
Now, that doesn’t mean you should bring out balloons and colorful wigs. It's just as easy to set the right tone from the start - and the best way to do that is to give credit where it's left.
Maintain consistency
Maintain consistency It is helpful to have a meeting at the same time every week so that your sales representatives get into the habit of blocking the same time on a weekly basis.
This will improve attendance, as your team will always know not to book more of that time unless absolutely necessary. If your team only has experienced salespeople, you can scale your sales meeting back and forth to give them more time to close deals.
How to Increase Customer Retention RatesSales
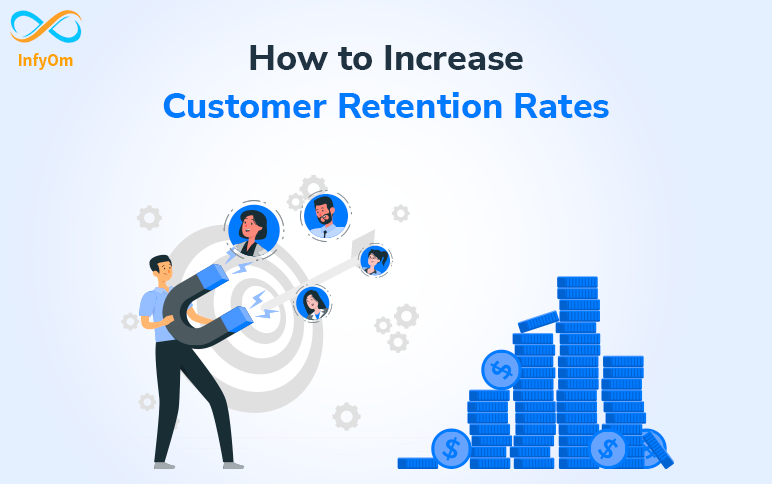
How to Increase Customer Retention RatesSales
Customer retention is the process of attracting repeat customers and preventing them from moving toward competitors. It is an important aspect of business strategy, and it can help businesses gain a competitive advantage.
The following ways to increase customer retention apply to virtually any type of business
Deliver more than you promised
The next step in the process is to deliver more than you promised - which means going beyond and beyond the call of duty and delivering to your customers the things they didn't expect. For example, you could offer a free bonus (such as a product, discount, or value-added) out of the blue, or anticipate a new customer's need and actively address it.
Meet your customers wherever they are
When you really understand your customers - that is, you know who they are, what they want from you, what their challenges are, and where they spend their time - you will reach them wherever they are. You can create the type of content they want and want (eg blog, video, social media) and then share it wherever they are (eg various websites, media channels, social platforms, etc.).
Good values build good relationships
Your company values are important to you. It should reflect your business processes, the quality of your products, and how you treat your customers. These things should make your values clear to your customers, but reminding them occasionally doesn't hurt.
Trust is the good relationship
Creating a brand that is easily relevant is the first step in building trust with your customers. Having something in common parental trust is the key to building a successful business, through a strong relationship and expansion.
Accept feedback
You never know what your customers really want until you ask. Take regular surveys and request feedback from all your customers. You never know what is missing in you - and what areas need improvement.
Follow up with your existing customers
High touch is the key to retaining the customer. The only unusual thing about personal follow-up is how little companies do it. Getting referrals from happy customers is easier than finding and converting a new business.
How To Increase Sales Through Digital MarketingSales
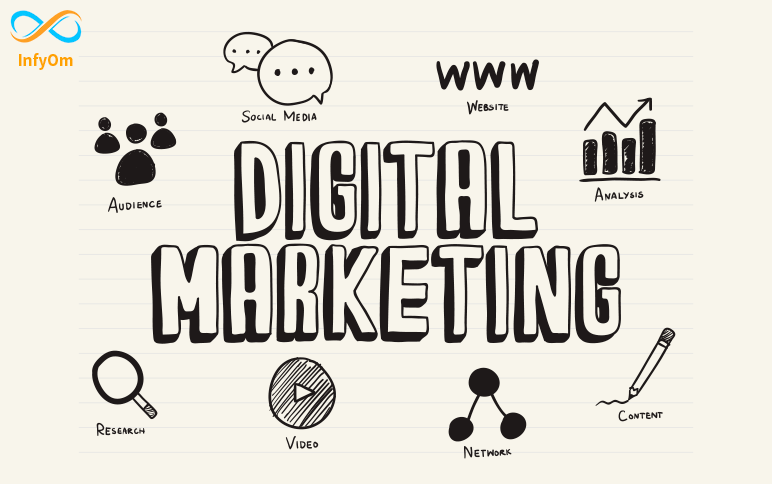
How To Increase Sales Through Digital MarketingSales
Introduction
Digital marketing can increase sales if we choose the best strategies.
Being an influential and well-known brand in your audience is something that is essential and positive.
Be available to your customers
75% of consumers expect to find a solution to their problem within five minutes of facing it. If you do not provide a solution, there is a good chance that they will not return to your business.
The online world is 24/7, so when you're not actively available to your customers, it's important that you set up a live chat, even if it's a bot.
Excessive communication with your customers
Transparency is key in the digital world. Trust is especially important where face-to-face communication is limited.
Building trust and then delivering on your promises is the key to enhancing the customer experience and ensuring that your brand's reputation is as strong as it can be to ultimately boost sales.
Offers and Discounts
We place some offers or discounts for our business during the festive season etc.
Clicking on Digital Marketing Pay will display advertisements of your business on various social media sites through advertising techniques. This ad will reach all the social media users so your sales will increase.
Use social networks
Social networks are very helpful in maintaining the reputation of your brand.
People like to have a unique experience with your brand on different social media channels. You need to choose the right channels for your business.
Create videos for YouTube
YouTube is an online video platform with millions of users and viewers every day. You should use these leading platforms as they can help your business grow through video views alone.
Videos can be made by you, your team, or (for more professional videos), videographer or photographer. Make sure your content is relevant to your audience or create videos specifically related to your business.