Laravel UPCItemDB Package
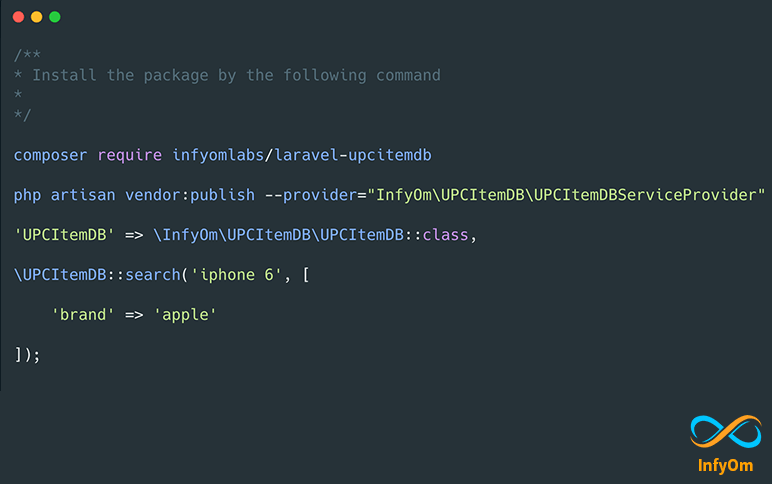
Announcing laravel UPCItemDB package to retrieve item details from the UPC code from…
How to Create Laravel Facade and Simplify Code
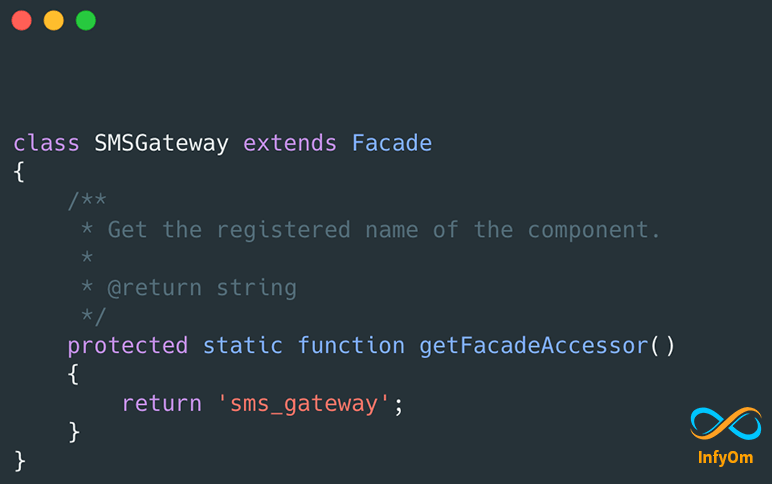
How to simplify laravel code by creating a laravel facade in a more structured and extensible…
Laravel App – Code Optimizations Tips
Tips about optimizing code and performance of Laravel Application.
How to display relationship data into Yajra DataTables with InfyOm Laravel Generator
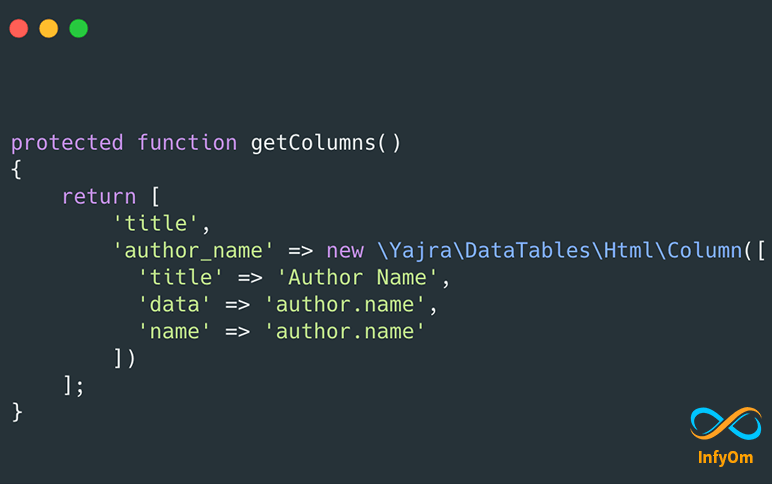
How to display relationship data into InfyOm Laravel Generator with Yajra…
How to use Datatables in InfyOm Laravel Generator
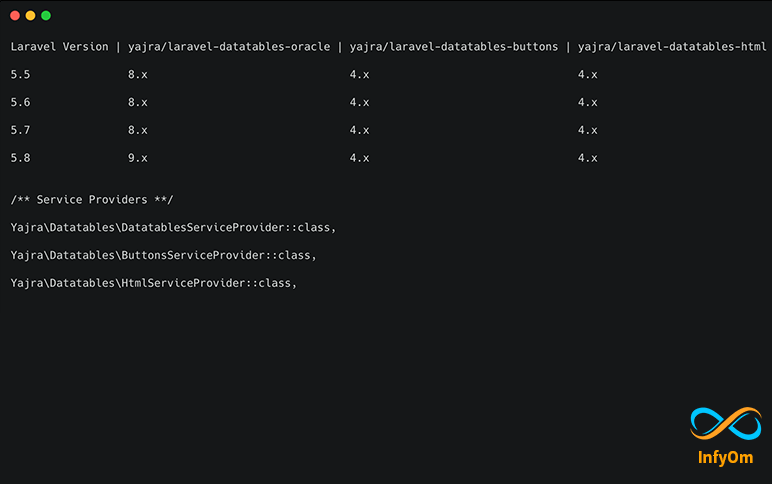
How to integrate jQuery Datatables with InfyOm Laravel Generator.
Introducing Laravel 5.8 support to InfyOm Laravel Generator
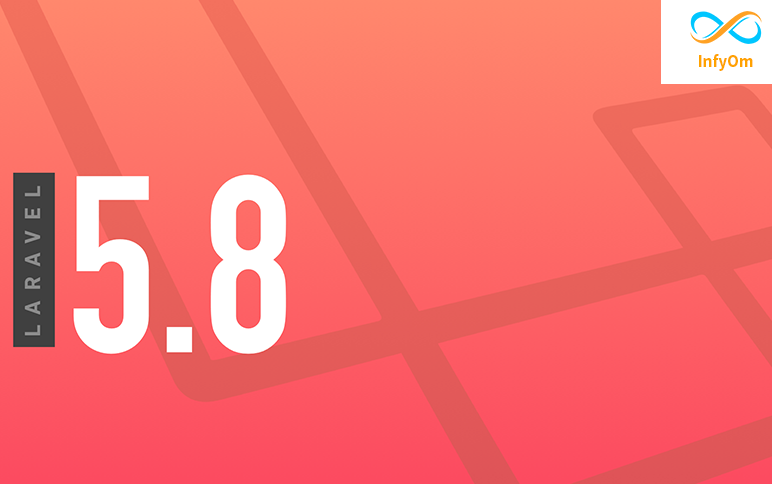
We have just added Laravel 5.8 version support to InfyOm Laravel Generator.
Integrate Amazon SNS into PHP Laravel to Send and Receive Messages
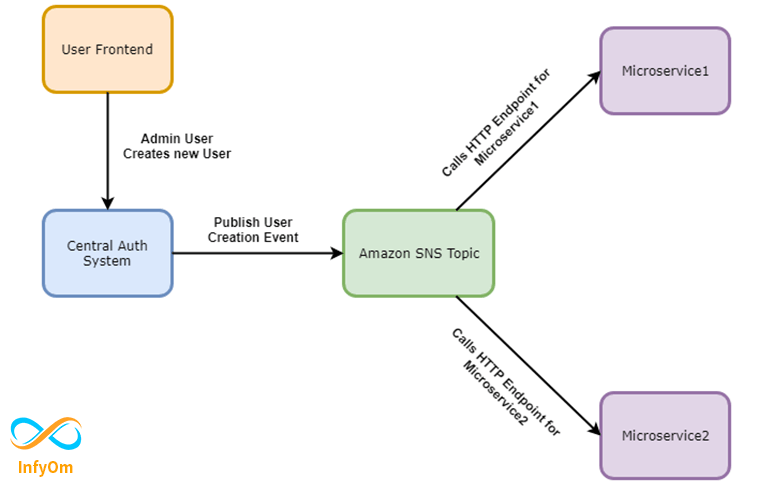
How to integrate Amazon SNS in the laravel app to send and receive messages via publishing and…
Multi Column Search box in Datatables with InfyOm Laravel Generator
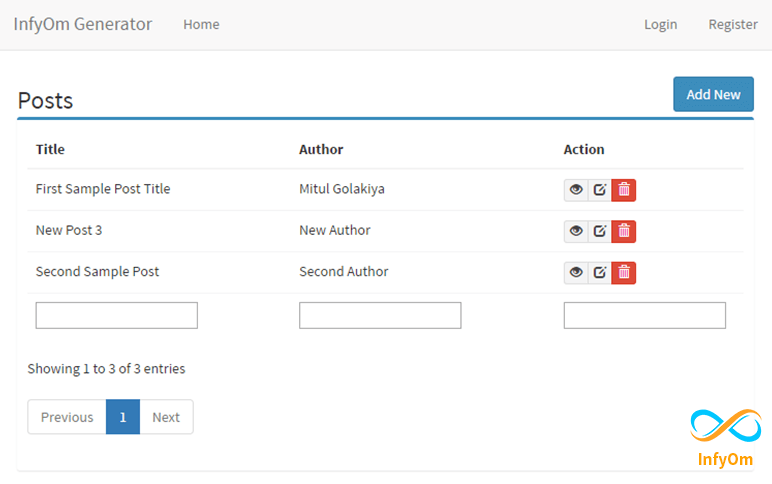
How to implement multi-column search in DataTables with InfyOm laravel generator…
How to Display Image in DataTable with yajra laravel-datatables with laravel generator
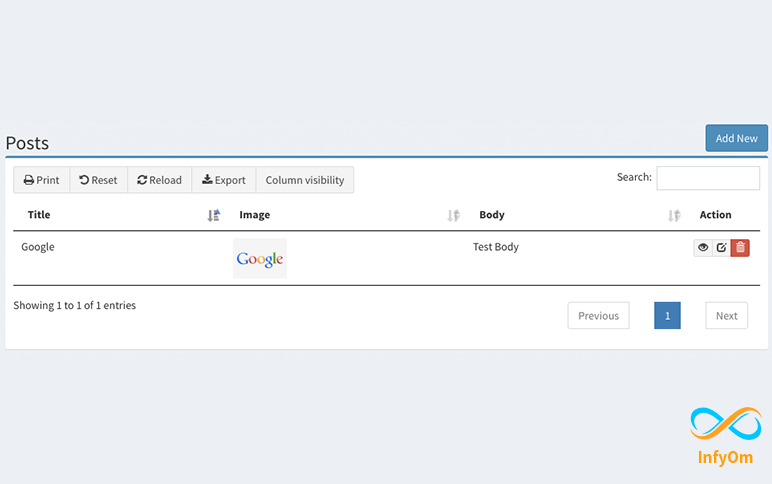
How to display an image in DataTable with laravel yajra datatable with InfyOm laravel…
24th Sep 2016 InfyOm Laravel Generator Release
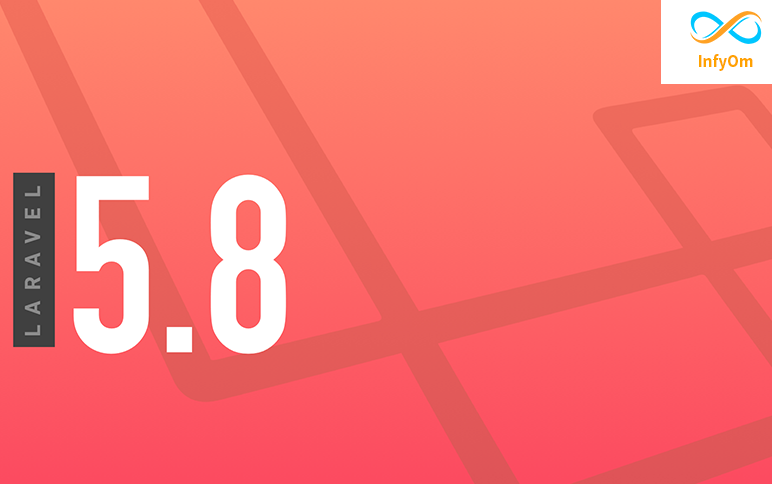
Release notes of InfyOm Laravel Generator on 24th September 2016.